top of page
Search
The Tech Platform
Nov 2, 2020
Quicksort example program in c++:
#include<iostream> #include<cstdlib> using namespace std; // Swapping two values. void swap(int *a, int *b) { int temp; temp = *a;...
The Tech Platform
Nov 2, 2020
Implementation of Quick Sort Algorithm in C:
# include <stdio.h> // to swap two numbers void swap(int* a, int* b) { int t = *a; *a = *b; *b = t; } int partition (int arr[], int...
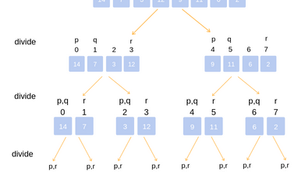
The Tech Platform
Nov 2, 2020
Merge Sort Algorithm
Merge sort is one of the most efficient sorting algorithms. It works on the principle of Divide and Conquer. Merge sort repeatedly breaks...
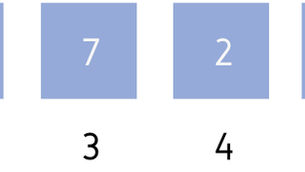
The Tech Platform
Nov 2, 2020
Selection Sort
Selection Sort is a simple comparison-based sorting algorithm that divides the input array into two parts: a sorted part and an unsorted...
The Tech Platform
Nov 2, 2020
Selection sort program in C++:
#include <iostream> #include <vector> using namespace std; int findMinIndex(vector<int> &A, int start) { int min_index = start; ...
The Tech Platform
Nov 2, 2020
Selection sort program in C:
#include <stdio.h> void swap(int *A, int i, int j) { int temp = A[i]; A[i] = A[j]; A[j] = temp; } int findMinIndex(int *A, int start,...
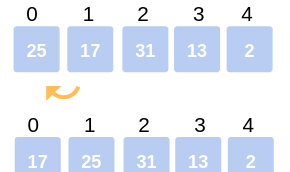
The Tech Platform
Nov 2, 2020
Insertion Sort Algorithm
Insertion sort is a simple sorting algorithm that builds the final sorted array one element at a time. It is an in-place and stable...
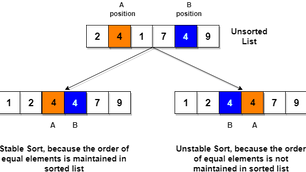
The Tech Platform
Nov 2, 2020
Insertion Sort Implementation in C++
#include <stdlib.h> #include <iostream> using namespace std; //member functions declaration void insertionSort(int arr[], int length);...
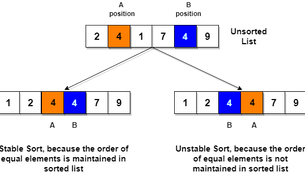
The Tech Platform
Nov 2, 2020
Insertion Sort Implementation In C
#include <stdio.h> #include <stdbool.h> #define MAX 7 //defining size of our array int intArray[MAX] = {4,6,3,2,1,9,7}; void...
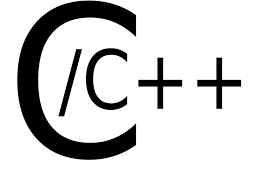
The Tech Platform
Oct 16, 2020
Best Programming Language You should know for CyberSecurity
Wh Programming Language is Important for Cybersecurity While it would be impossible for any one person to fill all the roles that make an...
bottom of page