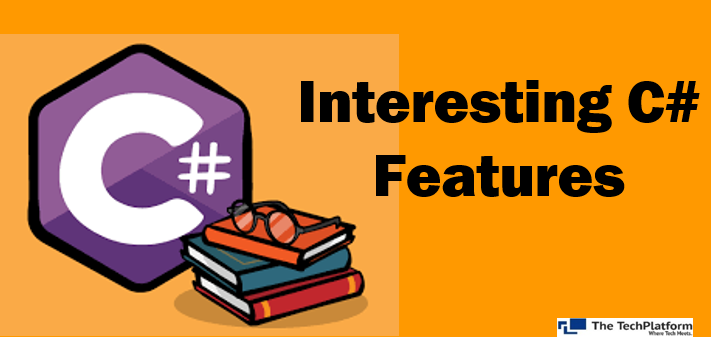
The ?? & ??= Operator
Use the “??” operator to set values conditionally from the left or right side.
int? x = null; // create a nullable type
int y = x ?? –1; // returns left side if null else right side
set conditionally in inverse (available in C# 8.0)
int? x = null; // create a nullable type
int y = -1 ?? x; // returns right side if null else left side
Volatile
The volatile keyword indicates that a field might be modified by multiple threads that are executing at the same time. The compiler, the runtime system, and even hardware may rearrange reads and writes to memory locations for performance reasons. Fields that are declared volatile are not subject to these optimizations. Adding the volatile modifier ensures that all threads will observe volatile writes performed by any other thread in the order in which they were performed. There is no guarantee of a single total ordering of volatile writes as seen from all threads of execution. ~Microsoft Docs
class VolatileTest
{
public volatile int sharedStorage;
public void Test(int _i)
{
sharedStorage = _i;
}
}
Collection Indexing Operators
public interface IActor
{
public string Name { get; set; }
public date BirthDate { get; set; }
public string TopMovie { get; set; }
public void InitializeActor(string Name, date BirthDate, string TopMovie)
{
this.Name = Name;
this.BirthDate = BirthDate;
this.TopMovie = TopMovie;
}
}IActor actor = new Actor();
actor.InitializeActor("Christian Bale", Date.Parse("2013-07-04"), "The Dark Knight");
Basic item indexing
Actor actor = actor[0];
Access items counting from the end of the collection.
Actor actor = actor[^1];
Access a range of a collection
var actors = actor[..4];
Source: Medium - Robby Boney
The Tech Platform
Comments