Every user expects websites to load quickly and search engines favor speedy pages in their rankings. The developers will optimize every aspect of our applications, from database queries to front-end rendering. The most important overlooked are the static assets-CSS files, JavaScript, and images the visual components of web pages. All these assets determine how fast the website loads and how responsive they are.
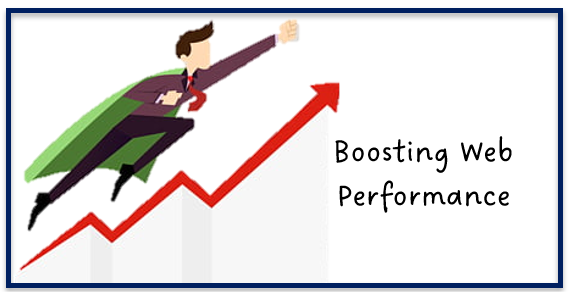
This article will help you explore a new tool for optimizing static asset delivery in ASP.NET Core: MapStaticAsset. What it is, why it matters, and how to integrate it in your project.
Static Asset Optimization
Static asset optimization improves the performance and efficiency of static resources on a website. These optimizations enhance user experience, reduce load times, and minimize bandwidth usage.
Optimizing the delivery of static assets is important for:
Page Load Speed: Faster-loading web pages enhance user experience. Optimized assets reduce initial load times, leading to improved performance and engagement.
Bandwidth Efficiency: Efficient asset delivery minimizes data transfer. Smaller file sizes reduce bandwidth consumption, benefiting both users and server costs.
SEO and Rankings: Search engines consider page speed as a ranking factor. Well-optimized assets contribute to better search engine visibility.
User Retention: Slow-loading pages frustrate users and increase bounce rates. Optimized assets keep visitors engaged and encourage longer sessions.
Here are some of the challenges you can face without proper optimization:
Additional Requests: Unoptimized assets result in multiple HTTP requests. Each request adds latency, impacting overall page load time.
Inefficient Data Transfer: Large asset files consume more bandwidth. Overloaded networks lead to slower loading, especially on mobile devices.
Serving Outdated Files: Browsers may serve cached, outdated assets. Users see stale content, affecting their experience without proper caching and version control.
Introducing MapStaticAssets
MapStaticAssets is a new middleware introduced in ASP.NET Core to enhance the delivery of static assets (such as CSS files, JavaScript scripts, and images) to web browsers. Its primary goal is asset serving, making web applications faster and more efficient.
The Problem It Addresses
Traditionally, developers relied on the UseStaticFiles middleware to serve static assets in ASP.NET Core applications. However, this approach had certain limitations:
Lack of Build-Time Compression:
UseStaticFiles did not provide build-time compression for assets.
During development, assets were served without any compression.
This resulted in larger file sizes transferred over the network, impacting page load times.
Inefficient Caching with ETags:
ETags (entity tags) are used for caching purposes. They allow browsers to determine whether a resource has changed since the last request.
UseStaticFiles did not offer content-based ETags. Instead, it relied on file modification timestamps.
As a result, browsers might redownload assets even when their content remains unchanged.
Lack of Fingerprinting:
Fingerprinting involves uniquely identifying assets to prevent serving outdated versions.
Without fingerprinting, browsers could cache and reuse old versions of assets, leading to inconsistencies after app updates.
Why Introduce MapStaticAssets?
The introduction of MapStaticAssets aims to address these limitations and provide a more robust solution for static asset delivery:
Build-Time Compression: MapStaticAssets compress assets during both development and publish time. During development, it uses gzip compression. During publish, it further compresses assets using both gzip and brotli. Smaller asset sizes reduce network transfer time and improve overall performance.
Content-Based ETags: MapStaticAssets calculates ETags based on the SHA-256 hash of asset content. When an asset’s content changes, its ETag changes accordingly. Efficient caching ensures that browsers redownload assets only when necessary, avoiding unnecessary network requests.
Fingerprinting Assets: By uniquely identifying assets, MapStaticAssets prevents browsers from reusing old versions. When an app is updated, the fingerprint changes, ensuring that clients always receive the latest assets.
Here is the difference between MapStatciAssets and UserStaticFiles in ASP.NET Core:
Aspect | UseStaticFiles | MapStaticAssets |
Purpose | Serves static files from the web root (e.g., CSS, JavaScript, images). | Optimizes static asset delivery. |
Configuration | Simple to set up with app.UseStaticFiles() middleware. | Requires app.MapStaticAssets() configuration. |
Compression | No build-time compression. | Provides build-time compression (gzip during development, gzip + brotli during publish). |
ETags | Uses file modification timestamps for caching. | Calculates content-based ETags (SHA-256 hash of content). |
Fingerprinting | No built-in fingerprinting. | Prevents serving outdated versions by uniquely identifying assets. |
Compatibility | Works with all UI frameworks (Blazor, Razor Pages, MVC). | Compatible with Blazor, Razor Pages, and MVC. |
How to Implementation MapStaticAssets in ASP.NET Core
First, ensure you have an ASP.NET Core project set up. If not, create a new one or use an existing project.
In your Program.cs file, configure MapStaticAssets by calling MapStaticAssets in the app’s request processing pipeline. This middleware performs the following tasks:
Sets the ETag and Last-Modified headers.
Sets caching headers.
Uses Caching Middleware.
When possible, serves compressed static assets.
Here’s an example snippet from Program.cs:
var builder = WebApplication.CreateBuilder(args);
// Other services and configurations...
if (!builder.Environment.IsDevelopment())
{
// Other production-related configurations...
}
app.UseHttpsRedirection();
app.UseStaticFiles(); // Enable serving static files
app.UseAuthorization();
// Other middleware configurations...
app.MapStaticAssets(); // Configure MapStaticAssets
app.Run();
In the above code
app.UseStaticFiles() enables serving static files from the wwwroot folder. You can reference these files in your HTML or other resources.
app.MapStaticAssets() configures MapStaticAssets to optimize asset delivery. It sets ETag and Last-Modified headers, applies caching, and serves compressed assets.
For example, if you have an image named MyImage.jpg in the wwwroot/images folder, you can use the following markup to display it:
<img src="~/images/MyImage.jpg" class="img" alt="My image" />
The tilde character ~ points to the web root.
Compatibility with UI Frameworks
Blazor: MapStaticAssets works seamlessly with Blazor applications. You can serve static assets efficiently, whether you’re building server-side Blazor or client-side Blazor (WebAssembly).
Razor Pages: Razor Pages also benefit from MapStaticAssets. It optimizes asset delivery for Razor Pages, making your web pages load faster.
MVC (Model-View-Controller): If you’re using the MVC pattern, MapStaticAssets integrates smoothly. Whether you’re building APIs or traditional web applications, it ensures efficient static asset serving.
Conclusion
By integrating MapStaticAsset into your ASP.NET Core projects, you unlock a host of benefits:
Speedier Page Loads: Compressed assets and efficient caching mechanisms lead to faster initial load times. Users appreciate snappy websites that respond promptly.
Bandwidth Savings: Smaller file sizes mean less data transfer. Reduced bandwidth consumption benefits both users and server costs.
SEO Boost: Search engines favor fast-loading pages. Well-optimized assets contribute to better search engine rankings.
User Satisfaction: Slow-loading pages frustrate visitors. Optimized assets keep users engaged and encourage longer sessions.
Comments