Sometimes we need to calculate the number of days between two dates. This can be done using the JavaScript programming language. JavaScript provides a math function Math.floor() method to calculate the days between two dates. In JavaScript, we have to use the date object for any calculation to define the date using new Date().
Code:
<html>
<h2>Calculate Number of days between two Given Dates:</h2>
<body>
<script>
var date1, date2;
//define two date object variables with dates inside it
date1 = new Date("07/03/2022");
date2 = new Date("11/05/2022");
//calculate time difference
var time_difference = date2.getTime() - date1.getTime();
//calculate days difference by dividing total milliseconds in a day
var days_difference = time_difference / (1000 * 60 * 60 * 24);
document.write("Dates are : <br>" +
"Date 1: " + date1+ " <br> Date 2:" + date2
+"<br>There are total "+ days_difference +" days");
</script>
</body>
</html>
Output:
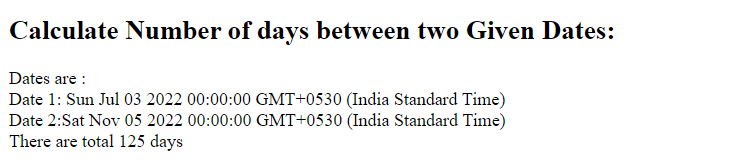
The Tech Platform
Комментарии