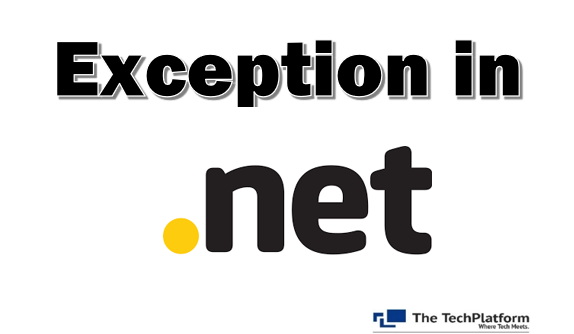
Exceptions are errors that occur during the runtime of a program. The advantage of using exceptions is that the program doesn’t terminate due to the occurrence of the exception. Whenever an exception is occurred the .NET runtime throws an object of specified type of Exception. The class ‘Exception’ is the base class of all the exceptions.
Exceptions can be thrown because of a fault in your code or in code that you call (such as a shared library), unavailable operating system resources, unexpected conditions that the runtime encounters (such as code that can't be verified), and so on. Your application can recover from some of these conditions, but not from others. Although you can recover from most application exceptions, you can't recover from most runtime exceptions.
Exceptions provide a way to transfer control from one part of a program to another. .Net exception handling is built upon four keywords - Try, Catch, Finally and Throw.
Try − A Try block identifies a block of code for which particular exceptions will be activated. It's followed by one or more Catch blocks.
Catch − A program catches an exception with an exception handler at the place in a program where you want to handle the problem. The Catch keyword indicates the catching of an exception.
Finally − The Finally block is used to execute a given set of statements, whether an exception is thrown or not thrown. For example, if you open a file, it must be closed whether an exception is raised or not.
Throw − A program throws an exception when a problem shows up. This is done using a Throw keyword.
Types of Exceptions:
In C#, There is one base class called Exception. It can be divided into two subparts.
System Exception.
Application Exception.
System Exceptions:
System exception is the base class for all built-in exception classes in .NET. There are many types of it. Common exceptions are -
IndexOutOfRange: Thrown by the runtime only when an array is indexed improperly.
NullReference : Thrown by the runtime only when a null object is referenced.
InvalidOperation: Thrown by methods when in an invalid state.
Argument: Base class for all argument exceptions.
ArgumentNull: Thrown by methods that do not allow an argument to be null.
ArgumentOutOfRange: Thrown by methods that verify that arguments are in a given range.
Application Exception:
It was recommended before, to derived if anyone wants to make their own custom exceptions based on a business rules violation. But now it was deprecated.
How to Throw Exception in .NET
You can explicitly throw an exception using the C# throw or the Visual Basic Throw statement. You can also throw a caught exception again using the throw statement. It is good coding practice to add information to an exception that is re-thrown to provide more information when debugging.
The following code example uses a try/catch block to catch a possible FileNotFoundException. Following the try block is a catch block that catches the FileNotFoundException and writes a message to the console if the data file is not found. The next statement is the throw statement that throws a new FileNotFoundException and adds text information to the exception.
using System;
using System.IO;
public class ProcessFile
{
public static void Main()
{
FileStream fs;
try
{
// Opens a text tile.
fs = new FileStream(@"C:\temp\data.txt", FileMode.Open);
var sr = new StreamReader(fs);
// A value is read from the file and output to the console.string line = sr.ReadLine();
Console.WriteLine(line);
}
catch(FileNotFoundException e)
{
Console.WriteLine($"[Data File Missing] {e}");
throw new FileNotFoundException(@"[data.txt not in c:\temp directory]", e);
}
finally
{
if (fs != null)
fs.Close();
}
}
}
The Tech Platform
Opmerkingen