Feature flags in ASP.NET Core allow us to change application behaviour i.e. enable or disable a specific functionality in the application at runtime without the need to make code changes or deploy a new version of the application. Feature flags are like a feature toggler that allows us to enable or disable a feature in an application in production.
Feature flags also allow you to gradually release a feature to the users i.e. first you can enable a new feature for just a few users of the application to check how it performs and once you are assured that it works as expected then you can enable this feature for remaining users either all at once or again gradually.
How to use Feature Flags?
1. Create an App Configuration store
STEP 1: To create a new App Configuration store, sign in to the Azure portal.
STEP 2: In the upper-left corner of the home page, select Create a resource.
STEP 3: In the Search services and marketplace box, enter App Configuration and select Enter.
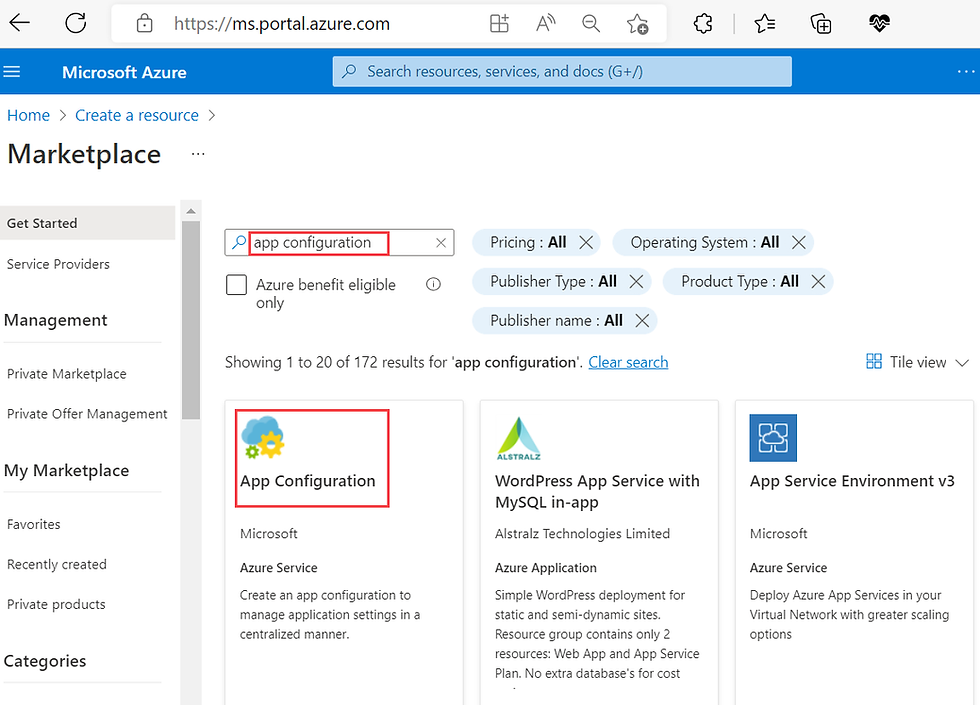
STEP 4: Select App Configuration from the search results, and then select Create.
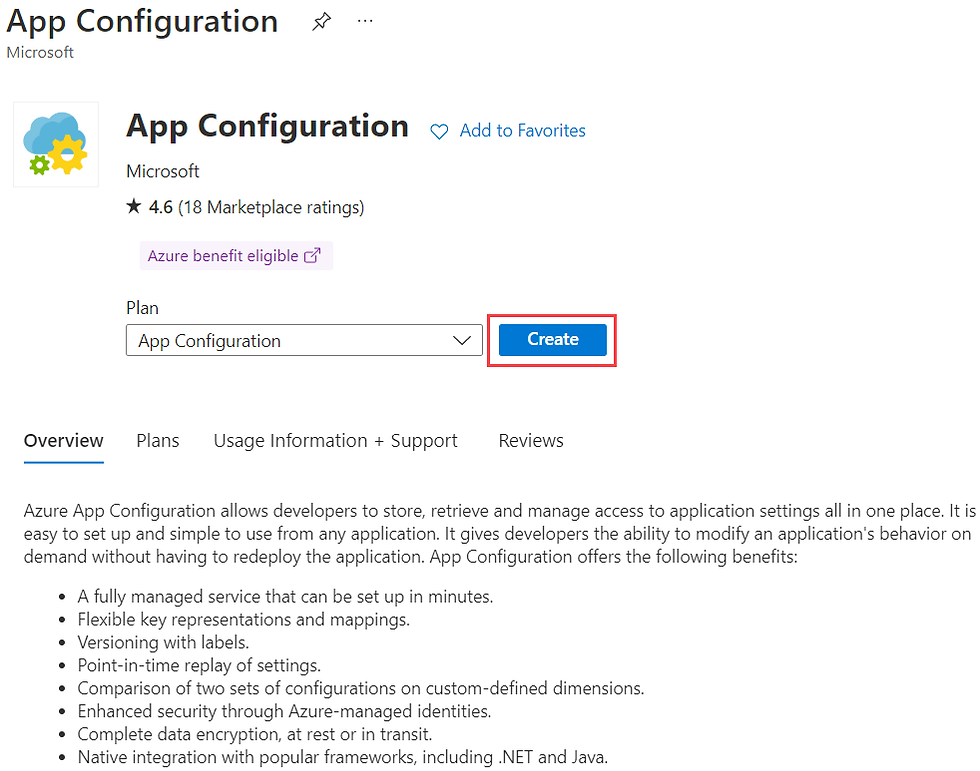
STEP 5: On the Create App Configuration pane, enter the following settings:
SETTING | SUGGESTED VALUE | DESCRIPTION |
Subscription | Your subscription | Select the Azure subscription that you want to use to test App Configuration. If your account has only one subscription, it's automatically selected and the Subscription list isn't displayed. |
Resource group | AppConfigTestResources | Select or create a resource group for your App Configuration store resource. This group is useful for organizing multiple resources that you might want to delete at the same time by deleting the resource group. For more information, see Use resource groups to manage your Azure resources. |
Resource name | Globally unique name | Enter a unique resource name to use for the App Configuration store resource. The name must be a string between 5 and 50 characters and contain only numbers, letters, and the - character. The name can't start or end with the - character. |
Location | Central US | Use Location to specify the geographic location in which your app configuration store is hosted. For the best performance, create the resource in the same region as other components of your application. |
Pricing tier | Free | Select the desired pricing tier. For more information, see the App Configuration pricing page. |
STEP 6: Select Review + create to validate your settings.
STEP 7: Select Create. The deployment might take a few minutes.
STEP 8: After the deployment finishes, go to the App Configuration resource. Select Settings > Access keys. Make a note of the primary read-only key connection string. You'll use this connection string later to configure your application to communicate with the App Configuration store that you created.
STEP 9: Select Operations > Feature manager > Add to add a feature flag called Beta.

Leave Label empty for now. Select Apply to save the new feature flag.
2. Create an ASP.NET Core web app
Use the .NET Core command-line interface (CLI) to create a new ASP.NET Core MVC project. The advantage of using the .NET Core CLI instead of Visual Studio is that the .NET Core CLI is available across the Windows, macOS, and Linux platforms.
Run the following command to create an ASP.NET Core MVC project in a new TestFeatureFlags folder:
dotnet new mvc --no-https --output TestFeatureFlags
3. Add Secret Manager
A tool called Secret Manager stores sensitive data for development work outside of your project tree. This approach helps prevent the accidental sharing of app secrets within source code. Complete the following steps to enable the use of Secret Manager in the ASP.NET Core project:
STEP 1: Navigate to the project's root directory, and run the following command to enable secrets storage in the project:
dotnet user-secrets init
STEP 2: A UserSecretsId element containing a GUID is added to the .csproj file:
<Project Sdk="Microsoft.NET.Sdk.Web">
<PropertyGroup>
<TargetFramework>net6.0</TargetFramework>
<Nullable>enable</Nullable>
<ImplicitUsings>enable</ImplicitUsings>
<UserSecretsId>8296b5b7-6db3-4ae9-a590-899ac642c0d7
</UserSecretsId>
</PropertyGroup>
</Project>
4. Connect to an App Configuration store
STEP 1: Install the Microsoft.Azure.AppConfiguration.AspNetCore and Microsoft.FeatureManagement.AspNetCore NuGet packages by running the following commands:
dotnet add package Microsoft.Azure.AppConfiguration.AspNetCore
dotnet add package Microsoft.FeatureManagement.AspNetCore
STEP 2: Run the following command in the same directory as the .csproj file. The command uses Secret Manager to store a secret named ConnectionStrings:AppConfig, which stores the connection string for your App Configuration store. Replace the <your_connection_string> placeholder with your App Configuration store's connection string. You can find the connection string under Access Keys in the Azure portal.
dotnet user-secrets set ConnectionStrings:AppConfig "<your_connection_string>"
Secret Manager is used only to test the web app locally. When the app is deployed to Azure App Service, use the Connection Strings application setting in App Service instead of Secret Manager to store the connection string.
Access this secret using the .NET Core Configuration API. A colon (:) works in the configuration name with the Configuration API on all supported platforms. For more information, see Configuration keys and values.
STEP 3: In Program.cs, update the CreateWebHostBuilder method to use App Configuration by calling the AddAzureAppConfiguration method.
Important CreateHostBuilder replaces CreateWebHostBuilder in .NET Core 3.x. Select the correct syntax based on your environment.
public static IHostBuilder CreateHostBuilder(string[] args) =>
Host.CreateDefaultBuilder(args)
.ConfigureWebHostDefaults(webBuilder =>
webBuilder.ConfigureAppConfiguration(config =>
{
var settings = config.Build();
var connection = settings.GetConnectionString("AppConfig");
config.AddAzureAppConfiguration(options =>
options.Connect(connection).UseFeatureFlags());
}).UseStartup<Startup>());
With the preceding change, the configuration provider for App Configuration has been registered with the .NET Core Configuration API.
STEP 4: In Startup.cs, add a reference to the .NET Core feature manager:
using Microsoft.FeatureManagement;
STEP 5: Update the Startup.ConfigureServices method to add feature flag support by calling the AddFeatureManagement method. Optionally, you can include any filter to be used with feature flags by calling AddFeatureFilter<FilterType>():
public void ConfigureServices(IServiceCollection services)
{
services.AddControllersWithViews();
services.AddFeatureManagement();
}
STEP 6: Add a MyFeatureFlags.cs file to the root project directory with the following code:
namespace TestFeatureFlags
{
public enum MyFeatureFlags
{
Beta
}
}
STEP 7: Add a BetaController.cs file to the Controllers directory with the following code:
using Microsoft.AspNetCore.Mvc;
using Microsoft.FeatureManagement;
using Microsoft.FeatureManagement.Mvc;
namespace TestFeatureFlags.Controllers
{
public class BetaController: Controller
{
private readonly IFeatureManager _featureManager;
public BetaController(IFeatureManagerSnapshot featureManager) =>
_featureManager = featureManager;
[FeatureGate(MyFeatureFlags.Beta)]
public IActionResult Index() => View();
}
}
STEP 8: In Views/_ViewImports.cshtml, register the feature manager Tag Helper using an @addTagHelper directive:
@addTagHelper *, Microsoft.FeatureManagement.AspNetCore
The preceding code allows the <feature> Tag Helper to be used in the project's .cshtml files.
STEP 9: Open _Layout.cshtml in the Views\Shared directory. Locate the <nav> bar code under <body> > <header>. Insert a new <feature> tag in between the Home and Privacy navbar items, as shown in the highlighted lines below.
//...
<nav class="navbar navbar-expand-sm navbar-toggleable-sm navbar-light bg-white border-bottom box-shadow mb-3">
<div class="container">
<a class="navbar-brand" asp-area="" asp-controller="Home"
asp-action="Index">TestFeatureFlags</a>
<button class="navbar-toggler" type="button" data-
toggle="collapse" data-target=".navbar-collapse" aria-
controls="navbarSupportedContent"aria-expanded="false"
aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="navbar-collapse collapse d-sm-inline-flex
flex-sm-row-reverse">
<ul class="navbar-nav flex-grow-1">
<li class="nav-item">
<a class="nav-link text-dark" asp-area=""
asp-controller="Home" asp-
action="Index">Home</a>
</li>
<feature name="Beta">
<li class="nav-item">
<a class="nav-link text-dark" asp-area=""
asp-controller="Beta" asp-
action="Index">Beta</a>
</li>
</feature>
<li class="nav-item">
<a class="nav-link text-dark" asp-area=""
asp-controller="Home" asp-
action="Privacy">Privacy</a>
</li>
</ul>
</div>
</div>
STEP 10: Create a Views/Beta directory and an Index.cshtml file containing the following markup:
@{
ViewData["Title"] = "Beta Home Page";
}
<h1>This is the beta website.</h1>
5. Build and run the app locally
STEP 1: To build the app by using the .NET Core CLI, run the following command in the command shell:
dotnet build
STEP 2: After the build successfully completes, run the following command to run the web app locally:
dotnet run
STEP 3: Open a browser window, and go to http://localhost:5000, which is the default URL for the web app hosted locally. If you're working in the Azure Cloud Shell, select the Web Preview button followed by Configure. When prompted, select port 5000.

Your browser should display a page similar to the image below.
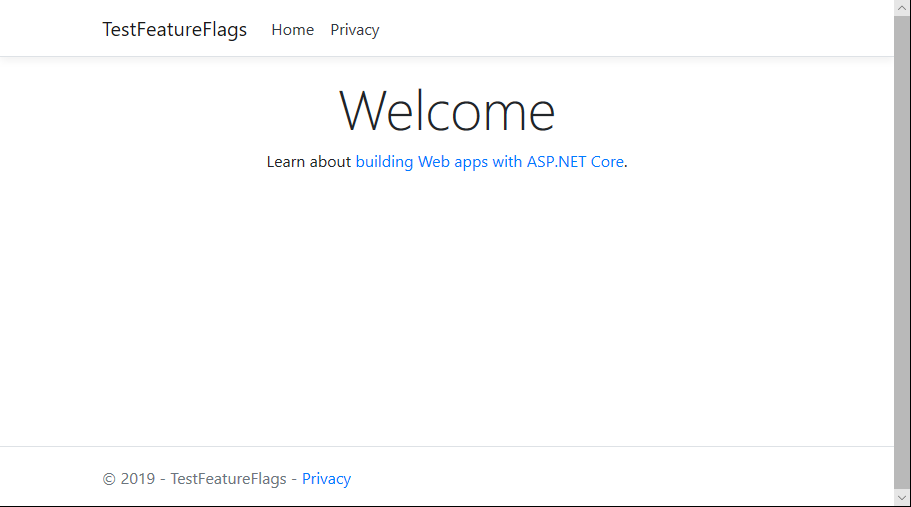
STEP 4: Sign in to the Azure portal. Select All resources, and select the App Configuration store instance that you created in the quickstart.
STEP 5: Select Feature manager.
STEP 6: Enable the Beta flag by selecting the checkbox under Enabled.
STEP 7: Return to the command shell. Cancel the running dotnet process by pressing Ctrl+C. Restart your app using dotnet run.
STEP 8: Refresh the browser page to see the new configuration settings.
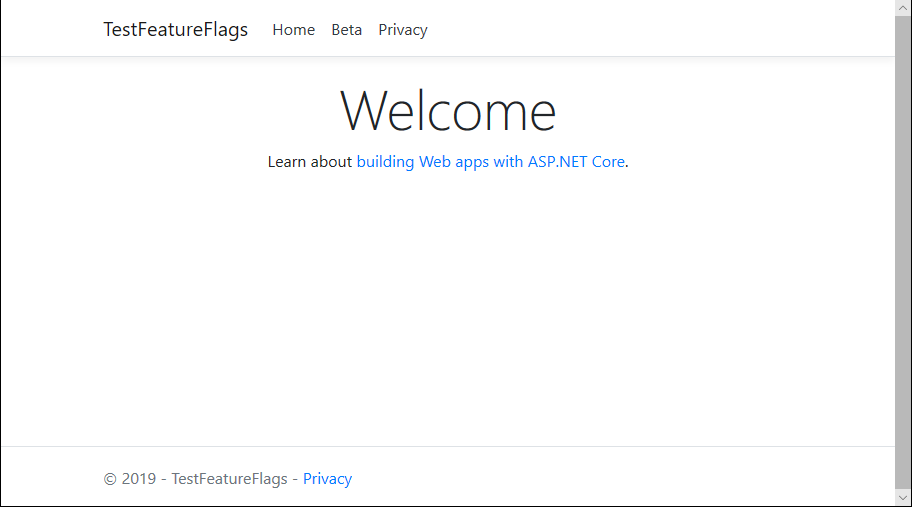
Feature Flags Drawbacks:
The feature flags in ASP.NET Core does add some dependency & complexity to the application as feature flags need to be managed and will also require maintenance.
In the long run, once a new feature is enabled for all the users and very well established on production such that it will never be reverted then in that case you need to consider removing that feature flag code and its configuration as well.
When you develop a feature with a feature flag option then there are additional testing needs for the application as you need to also test that feature is working as per the feature flag logic added to the application
Resource: Microsoft
The Tech Platform
Comentários