The code given below generates Pie Chart with static data.
<script type="text/javascript" src="https://code.jquery.com/jquery-3.0.0.min.js" >
</script>
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.0.0/jquery.min.js" ></script>
<script type="text/javascript" src="https://www.gstatic.com/charts/loader.js" ></script>
<head>
<script type="text/javascript">
google.charts.load("current", {packages:["corechart"]});
google.charts.setOnLoadCallback(drawChart);
$(document).ready(function() {
drawChart();
});
});
function drawChart() {
// Create and populate the data table.
var data = google.visualization.arrayToDataTable([
['Problems', 'Hours per Day'],
['ACMV', '0'],
['Carpentry & Hot works', '367'],
['Electrical', '83.55'],
['General', '63.1'],
['MHS','0'],
['Sanitation', '0'],
]);
var options = {
title: 'Facilities Work Order February',
is3D: true,
};
// Create and draw the visualization.
new google.visualization.PieChart(
document.getElementById('my_chart')).draw(data, options);
}
</script>
</head>
<body>
<div class="container">
<div class="row">
<div id="my_chart" style="width: 500px; height: 300px">
</div>
</div>
</div>
</body>
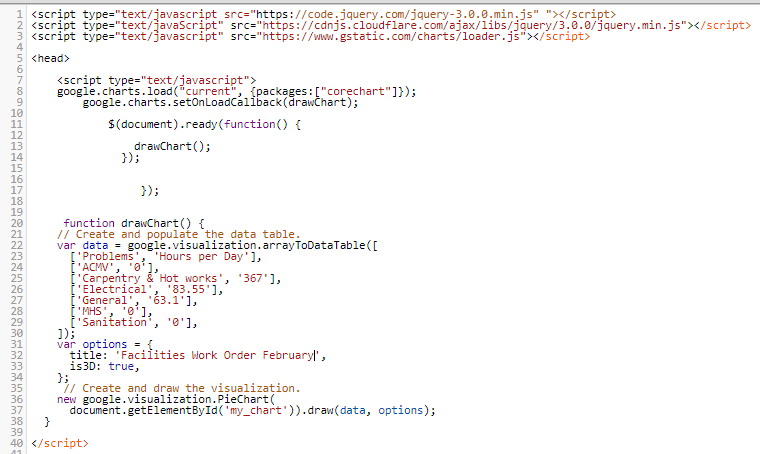
Google Pie chart in SharePoint online
I have hardcoded the value in the above code, but we can also call REST services to fetch the data from SharePoint Online list which I posted in below code.
<script type="text/javascript">
jQuery(document).ready(function () {
var testdata = [];
var URL="_spPageContextInfo.webAbsoluteUrl + "/_api/Web/Lists/GetByTitle('ListName')/Items?Selected=ID"
jQuery.ajax({
url: URL,
type: "GET",
headers: { "Accept": "application/json" },
success: function (data) {
//script to build UI HERE
var results = JSON.parse(data[1]);
jQuery.each(results,function (key, item) {
//You will get your data from REST API
});
var chart= new google.visualization.PieChart(document.getElementById('my_chart')).draw(data, options);
},
error: function (data) {
//output error HERE
alert(data.statusText);
}
});
});
</script>

Google Pie chart in SharePoint online
Integrate Google pie chart SharePoint Online
Once we have the code ready, we can add the JavaScript code inside a content editor web part or a script editor web part.
Here we will add the code inside a Script editor web part in a web part page.
Step-1: Go to your SharePoint web part page.

Script Editor Web part in SharePoint online
Step-2: Edit the web part page and add the ‘Script Editor’ to the page by following the below instructions (refer to the below screenshot).
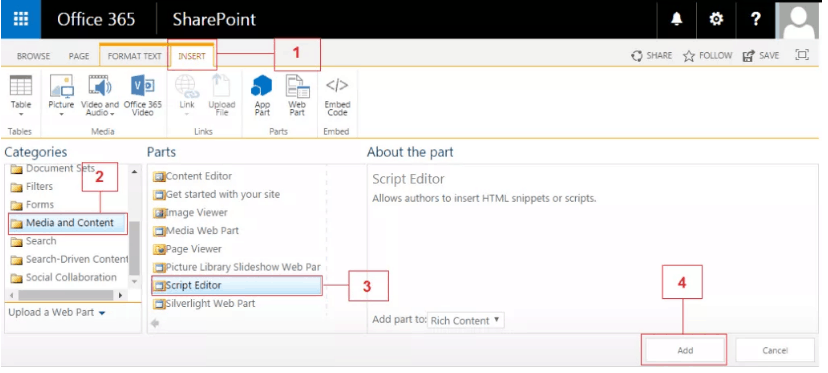
Script Editor Web part SharePoint online
Step-3: Click on the ‘Edit Web Part’ option as shown below.
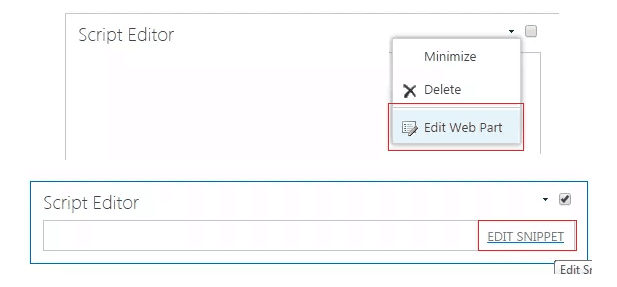
Script Editor Web parts SharePoint online
Step-4: Copy and paste the code snippet in the ‘Web Part’ which I have written on top and click on the ‘Insert’ button and save the page.
Step-5: Once you click on OK, you will get an output like Pie charts.
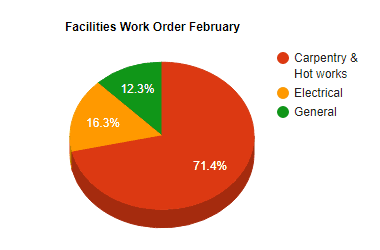
Google Pie chart in SharePoint online using JavaScript
Source: Paper.li
The Tech Platform
Comments