When I ask people to create singleton class they would happily create with following implementation.
class SecuritySingleton
{
public string Name { get; set; }
public bool IsAdmin { get; set; }
private SecuritySingleton()
{
}
# region Object Creation
private static Security Singleton _singleton;
public static Security Singleton GetInstance()
{
if (_singleton == null)
{
_singleton = new SecuritySingleton();
}
return _singleton;
}
# endregion
# region Public methods
public string GetNameWithAdminStatus()
{
if (IsAdmin)
{
return $"{Name} is an admin";
}
return $"{Name} is not an admin";
}
# endregion
}
Now imagine a scenario where you want to create single instance of class which is part of third party library or a class which is part of library which you can’t modify. In such scenario how would you create single instance of such classes.
In this tutorial I am going to explain how we can create single instance of such classes.
Also when we carefully examine the above code we find that this class is also responsible of creating the its own object other than the functionality that it provides. so it is also violating single responsibility.
So let’s get started by removing the instance creation part so that it become simple class to mimic as if it is coming from third party library.

Modified class with instance creation
Now this is a simple class and we can create as many instance as we want.
But wait.. that’s not what we wanted.
we wanted to have a single instance.
Now let’s deal with single instance creation part.
Create a generic singleton class like below
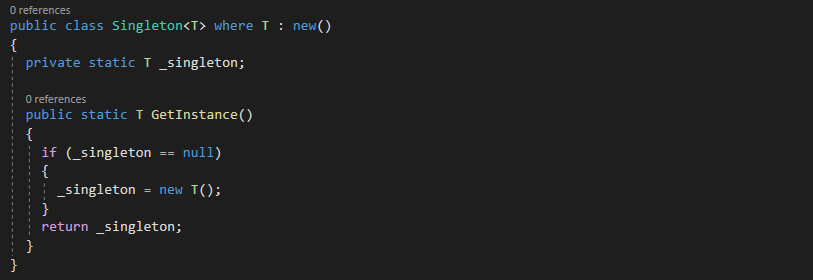
Generic singleton class
Now just create the object like below wherever needed. also notice(in the commented code) the changes from the time when object creation was part of same of class, it is just minimum changes from the consumer side.
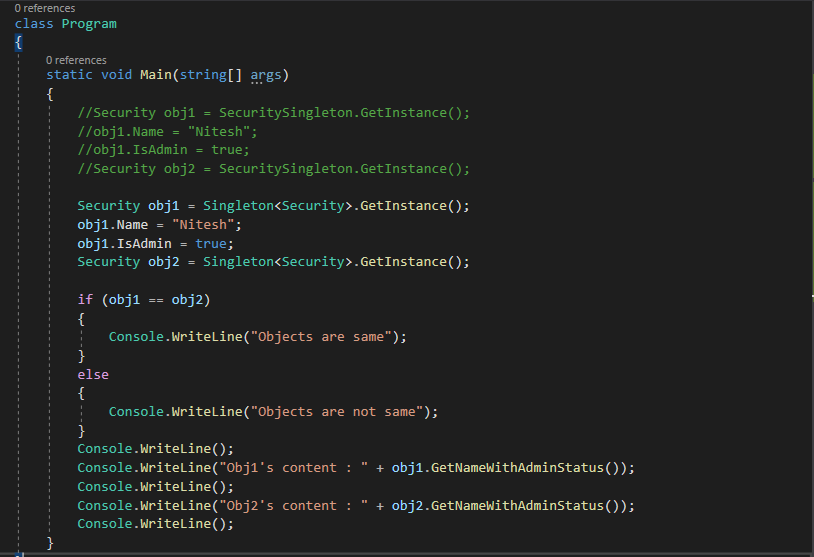
Main program
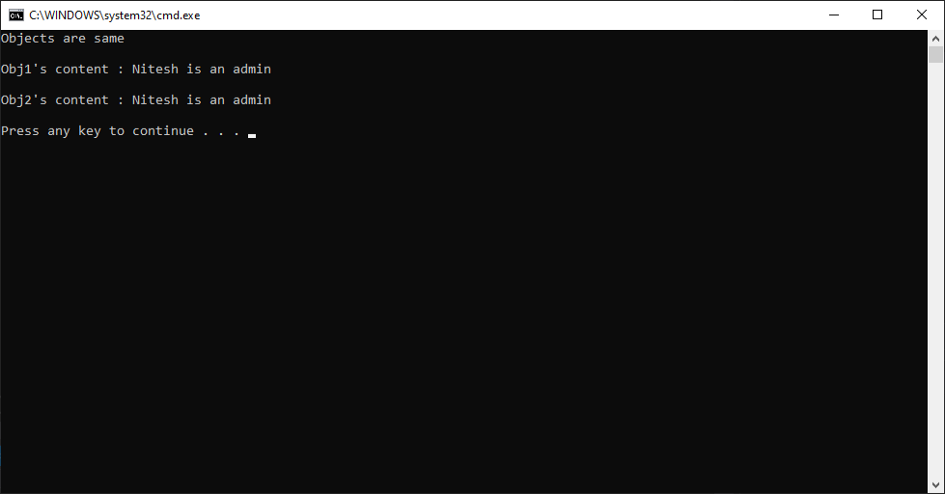
Result screen
As you can see in the result, both objects are same and also having same content.
Now I can create singleton for any class in my code without even modifying it.
Summary
In this tutorial, you have seen how easily we can create singleton for classes which is third party or you don’t have control to modify it.
Also as a byproduct, we have achieved single responsibility by removing the instance creation code.
Benefit of separating the instance creation is that we can create single instance of any class using this generic implementation and also Unit testing would be easy for those classes.
Source: Medium - Nitesh Singhal
The Tech Platform
Kommentare