To check whether a number is prime or not, we can write a function and then iterate through the first 100 numbers (1 to 100), printing only those that pass the prime test.
In Java, a number is considered prime if it is not divisible by any number other than itself, such as 2, 3, or 5. The number 1 is not counted as a prime number, so the lowest prime number is 2. To determine if a number is prime, one simple approach is to loop from 2 to the number itself and check if it is divisible by any number in between.
This check can be done using the modulus operator in Java, which returns zero if a number is perfectly divisible by another number. If the number being checked is not divisible by any number, it is considered a prime number; otherwise, it is not.
However, this logic can be optimized further by looping only up to the square root of the number instead of the number itself. This optimization is based on the fact that if a number is not divisible by any numbers up to its square root, it will not be divisible by any numbers greater than its square root. This optimization improves the performance of the Java program, especially when checking large prime numbers.
Here is a list of all the prime numbers between 1 and 100:
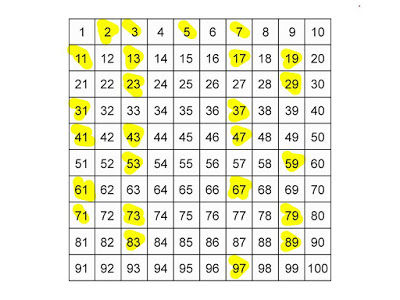
Here is a Java program that print prime numbers from 1 to 100 in java:
public class PrimeNumberGenerator
{
public static void main(String[] args) {
System.out.println("Prime numbers from 1 to 100:");
for (inti=2; i <= 100; i++) {
if (isPrime(i)) {
System.out.println(i);
}
}
}
public static boolean isPrime(int num)
{
if (num < 2) {
return false;
}
for (inti=2; i <= Math.sqrt(num); i++) {
if (num % i == 0) {
return false;
}
}
return true;
}
}
Output:
Prime numbers from 1 to 100
2
3
5
7
11
13
17
19
23
29
31
37
41
43
47
53
59
61
67
71
73
79
83
89
97
In this program, we have a main method that loops from 2 to 100 and checks if each number is prime by calling the isPrime method. The isPrime method checks if a number is divisible by any number from 2 to its square root. If it is divisible by any number, it returns false, indicating that the number is not prime. Otherwise, it returns true.
By using this program, we can generate and print all the prime numbers between 1 and 100.
Comments