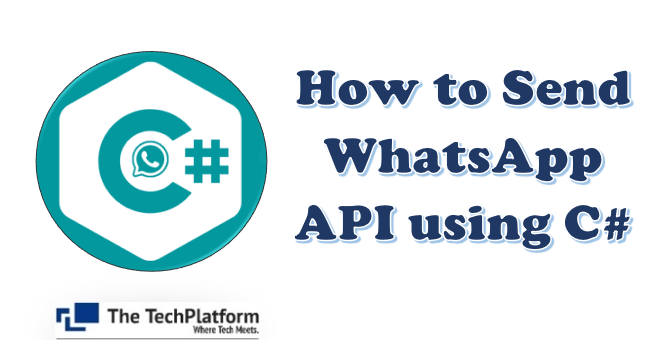
Get Started with Whatsapp API
Ultramsg account is required to run examples. Create an Account if you don’t have one.
go to your instance or Create one if you haven’t already.
Scan Qr and make sure that instance Auth Status: authenticated
Start sending Messages
Introduction
In this article, we will create simple examples to send messages via WhatsApp API using c#.
Initially, you must make sure that the RestSharp library is ready Because we will use this library In this article to send messages to the Ultramsg Gateway.
quick Example for WhatsApp API using C#
using System;
using RestSharp;public class Program
{
public static void Main()
{
///////////
var client =
new RsestClient("https://api.ultramsg.com/instance950/messages/chat");
var request = new RestRequest(Method.POST);
request.AddHeader("content-type", "application/x-www-form-urlencoded");
request
.AddParameter("undefined",
"token=95041eyo9eqixrsi&to=14155552671&body=WhatsApp API on UltraMsg.com works good&priority=10&referenceId=",
ParameterType.RequestBody);
IRestResponse response = client.Execute(request); ///////////
Console.WriteLine(response.Content);
}
}
When the previous code is executed, and if the message is sent successfully, the response will be like this:
{"sent":"true","message":"ok","id":497}
Send Image
var client = new RestClient("https://api.ultramsg.com/instance950/messages/image");
var request = new RestRequest(Method.POST);
request.AddHeader("content-type", "application/x-www-form-urlencoded");
request.AddParameter("undefined", "token=95041eyo9eqixrsi&to=14155552671&image=https://file-example.s3-accelerate.amazonaws.com/images/test.jpg&caption=image Caption&referenceId=", ParameterType.RequestBody);
IRestResponse response = client.Execute(request);
Send Document
var client = new RestClient("https://api.ultramsg.com/instance950/messages/document");
var request = new RestRequest(Method.POST);
request.AddHeader("content-type", "application/x-www-form-urlencoded");
request.AddParameter("undefined", "token=95041eyo9eqixrsi&to=14155552671&filename=hello.pdf&document=https://file-example.s3-accelerate.amazonaws.com/documents/cv.pdf&referenceId=", ParameterType.RequestBody);
IRestResponse response = client.Execute(request);
Send Audio
var client = new RestClient("https://api.ultramsg.com/instance950/messages/audio");
var request = new RestRequest(Method.POST);
request.AddHeader("content-type", "application/x-www-form-urlencoded");
request.AddParameter("undefined", "token=95041eyo9eqixrsi&to=14155552671&audio=https://file-example.s3-accelerate.amazonaws.com/audio/2.mp3&referenceId=", ParameterType.RequestBody);
IRestResponse response = client.Execute(request);
Send Voice
var client = new RestClient("https://api.ultramsg.com/instance950/messages/voice");
var request = new RestRequest(Method.POST);
request.AddHeader("content-type", "application/x-www-form-urlencoded");
request.AddParameter("undefined", "token=95041eyo9eqixrsi&to=14155552671&audio=https://file-example.s3-accelerate.amazonaws.com/voice/oog_example.ogg&referenceId=", ParameterType.RequestBody);
IRestResponse response = client.Execute(request);
Send Video
var client = new RestClient("https://api.ultramsg.com/instance950/messages/video");
var request = new RestRequest(Method.POST);
request.AddHeader("content-type", "application/x-www-form-urlencoded");
request.AddParameter("undefined", "token=95041eyo9eqixrsi&to=14155552671&video=https://file-example.s3-accelerate.amazonaws.com/video/test.mp4&referenceId=", ParameterType.RequestBody);
IRestResponse response = client.Execute(request);
Send Link
var client = new RestClient("https://api.ultramsg.com/instance950/messages/link");
var request = new RestRequest(Method.POST);
request.AddHeader("content-type", "application/x-www-form-urlencoded");
request.AddParameter("undefined", "token=95041eyo9eqixrsi&to=14155552671&link=https://en.wikipedia.org/wiki/COVID-19&referenceId=", ParameterType.RequestBody);
IRestResponse response = client.Execute(request);
Send Contact
var client = new RestClient("https://api.ultramsg.com/instance950/messages/contact");
var request = new RestRequest(Method.POST);
request.AddHeader("content-type", "application/x-www-form-urlencoded");
request.AddParameter("undefined", "token=95041eyo9eqixrsi&to=14155552671&contact=14000000001@c.us&referenceId=", ParameterType.RequestBody);
IRestResponse response = client.Execute(request);
Send Location
var client = new RestClient("https://api.ultramsg.com/instance950/messages/location");
var request = new RestRequest(Method.POST);
request.AddHeader("content-type", "application/x-www-form-urlencoded");
request.AddParameter("undefined", "token=1415555267141eyo9eqixrsi&to=14155552671&address=ABC company \n Sixth floor , office 38&lat=25.197197&lng=55.2721877&referenceId=", ParameterType.RequestBody);
IRestResponse response = client.Execute(request);
Send Vcard
var client = new RestClient("https://api.ultramsg.com/instance950/messages/vcard");
var request = new RestRequest(Method.POST);
request.AddHeader("content-type", "application/x-www-form-urlencoded");
request.AddParameter("undefined", "token=95041eyo9eqixrsi&to=14155552671&vcard= BEGIN:VCARD\nVERSION:3.0\nN:lastname;firstname\nFN:firstname lastname\nTEL;TYPE=CELL;waid=14000000001:14000000002\nNICKNAME:nickname\nBDAY:01.01.1987\nX-GENDER:M\nNOTE:note\nADR;TYPE=home:;;;;;;\nADR;TYPE=work_:;;;;;;\nEND:VCARD&referenceId=", ParameterType.RequestBody);
IRestResponse response = client.Execute(request);
finally, you can see Full Whatsapp API Documentation and FAQ.
Source: Medium - Ultramsg
The Tech Platform
Comments