A right join in C# LINQ is a type of join that returns each element of the second (right) data source, even if it has no correlated elements in the first data source. However, LINQ only supports left outer joins, which are the opposite of right joins. In this article, we will learn How to use Right Join in C# LINQ.
You can use LINQ to perform a right join by swapping the tables and doing a left join, or by calling the DefaultIfEmpty method on the results of a group join.
Before going further you may be interested in the following articles.
How to use Right Join in C# LINQ
Follow the below steps to use Right Join in C# LINQ:
STEP 1: Define the data sources that you want to join. For example, you can use two collections of objects, such as employees and departments.
STEP 2: Use the join keyword to perform a group join between the data sources based on a common key. The key must be of the same type and have equal values for matching elements. For example, you can join employees and departments based on the department ID.
STEP 3: Use the on keyword to specify the key for each data source. The key can be a property of the element or a value derived from the element. For example, you can use e.DepartmentId and d.Id as the keys for employees and departments respectively.
STEP 4: Use the equals keyword to compare the keys for equality. The keys must implement IEquatable<T> or IComparable<T>. For example, you can use an integer as the key type.
STEP 5: Use the into keyword to create a new identifier for each group. The identifier represents an IGrouping<TKey, TElement> object that contains a key and a sequence of elements that match that key. For example, you can use into ed to create a new identifier for each group.
STEP 6: Use the DefaultIfEmpty method on each group to perform a right join. This method returns a collection that contains a single default value if the group is empty, which means there are no matching elements from the left data source. For example, you can use DefaultIfEmpty to return a null value for departments that have no employees.
STEP 7: Use the select keyword to project the results into a new form. You can use anonymous types or named types to create the output. You can also access both data sources in the projection by using identifiers such as e and d. For example, you can select the department name and employee name for each pair.
Here is an example to use right join in C# LINQ:
csharpCopy code
// Define the data sourcesvar employees = new List<Employee>
{
new Employee { Id = 1, Name = "Alice", DepartmentId = 1 },
new Employee { Id = 2, Name = "Bob", DepartmentId = 2 },
new Employee { Id = 3, Name = "Charlie", DepartmentId = null },
new Employee { Id = 4, Name = "David", DepartmentId = 3 },
new Employee { Id = 5, Name = "Eve", DepartmentId = 2 }
};
var departments = new List<Department>
{
new Department { Id = 1, Name = "Sales" },
new Department { Id = 2, Name = "Marketing" },
new Department { Id = 3, Name = "IT" },
new Department { Id = 4, Name = "HR" }
};
// Perform right join using LINQvar query = from d in departments
join e in employees
on d.Id equals e.DepartmentId into ed
from e in ed.DefaultIfEmpty()
select new
{
DepartmentName = d.Name,
EmployeeName = e?.Name ?? "None"
};
// Display the resultsforeach (var result in query)
{
Console.WriteLine($"Department {result.DepartmentName} has employee {result.EmployeeName}");
}
Output:
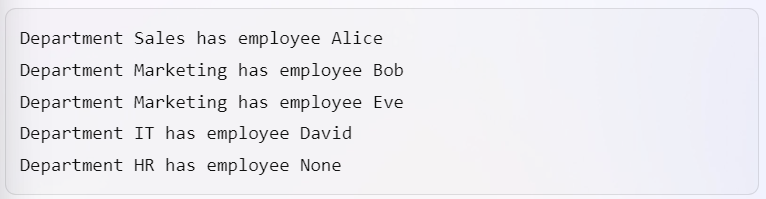
In this code, we first define two data sources: employees and departments. We then perform a right join using the LINQ join operator, which combines the elements of two sequences based on a common key. In this case, we join departments with employees based on their respective Id and DepartmentId properties.
To perform a right join, we use the DefaultIfEmpty method to return a default value when there is no matching element in the left sequence. In this case, we want to return the string "None" when there is no matching employee for a department.
Finally, we use the select operator to create a new anonymous type that contains the DepartmentName and EmployeeName properties. We then iterate over the query results and display them using Console.WriteLine.
Comments