In this article, we will find the Factorial of a Number in Java using 4 methods: For loop, While loop, Recursion, and BigInteger.
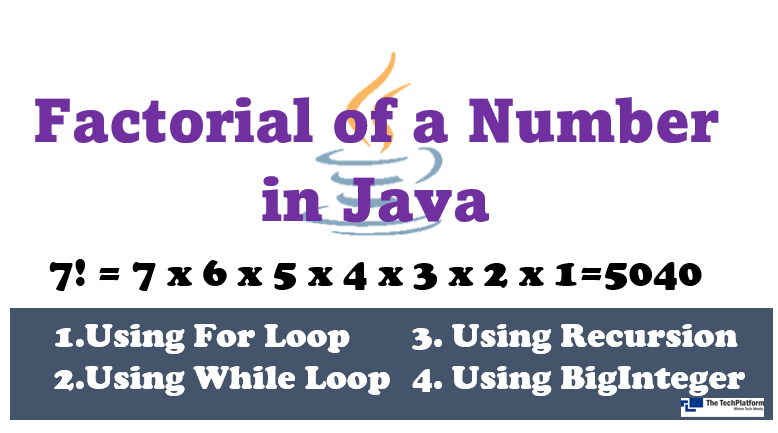
Factorial of a Number
The Factorial of a positive integer is the multiplication of all positive integers smaller than or equal to n. For example factorial of 6 is 6 x 5 x 4 x 3 x 2 x 1 = 720.
Algorithm:
Step 1: Start
Step 2: Read the number n
Step 3: [Initialize]
i=1, fact=1
Step 4: Repeat step 4 through 6 until i=n
Step 5: fact=fact*i
Step 6: i=i+1
Step 7: Print the fact
Step 8: Stop
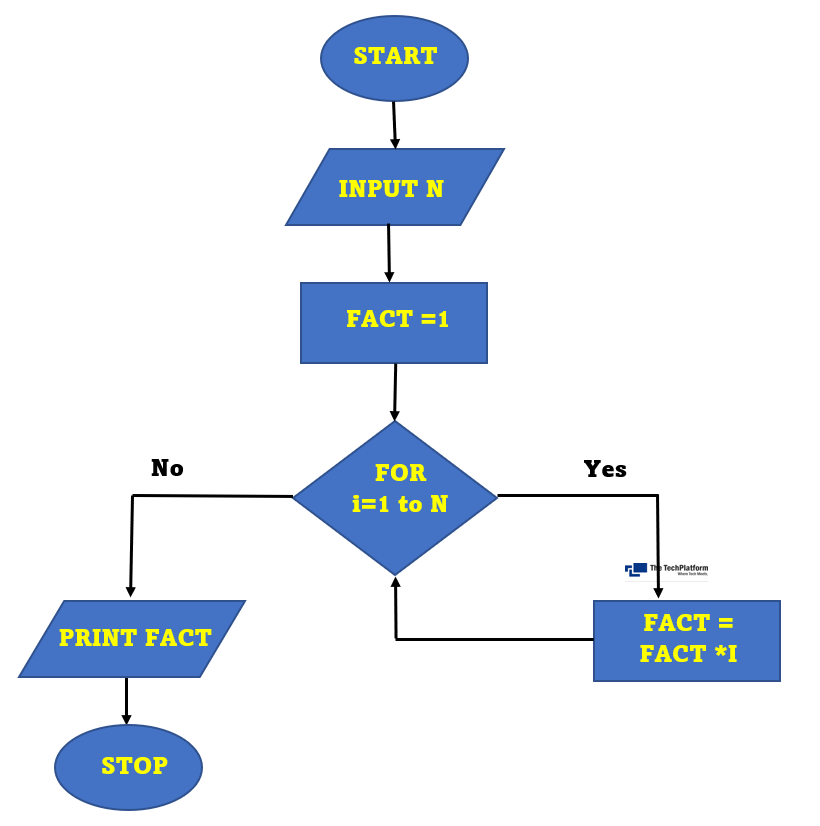
Method 1: Using For loop
Code:
class FactorialExample
{
public static void main(String args[])
{
int i,fact=1;
int number = 7; //It is the number to calculate factorial
for(i=1;i<=number;i++)
{
fact=fact*i;
}
System.out.println("Factorial of "+number+" is: "+fact);
}
}
Output:

Method 2: Using while loop
Code:
public class Factorial {
public static void main(String[] args) {
int num = 8, i = 1;
long factorial = 1;
while(i <= num)
{
factorial *= i;
i++;
}
System.out.printf("Factorial of %d = %d", num, factorial);
}
}
Output:

Method 3: Using Recursion
Code:
class FactorialExample2
{
static int factorial(int n)
{
if (n == 0)
return 1;
else
return(n * factorial(n-1));
}
public static void main(String args[])
{
int i,fact=1;
int number = 5;//It is the number to calculate factorial
fact = factorial(number);
System.out.println("Factorial of "+number+" is: "+fact);
}
}
Output:

Method 4: Using BigInteger
Code:
import java.math.BigInteger;
public class Factorial {
public static void main(String[] args) {
int num = 25;
BigInteger factorial = BigInteger.ONE;
for(int i = 1; i <= num; ++i)
{
// factorial = factorial * i;
factorial = factorial.multiply(BigInteger.valueOf(i));
}
System.out.printf("Factorial of %d = %d", num, factorial);
}
}
Output:

The Tech Platform
コメント