Python, a versatile and widely-used programming language, incorporates NULL into its syntax through the use of the None keyword. None plays a pivotal role in various aspects of Python programming, from initializing variables to indicating the absence of function return values. It allows developers to elegantly handle scenarios where data may be missing or undefined without causing unexpected errors.
In this article, we will explore the multifaceted world of NULL in Python. We'll cover the basics of using None, how to incorporate it into conditional statements and functions and examine its significance in Python's overall design philosophy. Whether you're a beginner learning Python or an experienced developer looking to deepen your understanding of NULL in Python, this guide will equip you with the knowledge and tools to navigate this critical concept effectively.
Table of Contents:
Techniques for Safely Handling NULL Values
Best Practices for Dealing with NULL Values
What is NULL in Python?
NULL in Python is a concept that represents the absence of a value or a missing or undefined data point. It's crucial in many programming languages because it allows developers to indicate when a variable or data structure doesn't hold a meaningful value. This is especially important when working with databases, user input, or handling exceptional cases in code. NULL in Python plays a vital role in preventing unexpected behavior and errors in programs.
Understanding 'None' in Python
Uses the concept of NULL in Python to represent the absence of a value. However, the equivalent of NULL n Python is represented by the None keyword. When a variable is assigned None, it signifies that the variable has no valid data or value associated with it. Python's None is used extensively for various purposes, such as initializing variables, indicating the absence of return values from functions, or as a placeholder.
Examples of Using 'None' in Python Code:
Below are the steps which show how to use 'None' in Python:
1. Initializing a Variable with None:
my_variable = None
In this example, my_variable is initialized with None, indicating that it does not currently hold any specific value.
2. Using None as a Default Function Return Value:
def find_element_in_list(element, my_list):
for item in my_list:
if item == element:
return item
return None # If the element is not found, return None.
Here, if the find_element_in_list function doesn't find the specified element in the list, it returns None to indicate that the element was not found.
3. Initializing an Empty List with None:
my_list = None
if not my_list:
my_list = [] # Initialize my_list as an empty list.
In this case, my_list is initially set to None, but it is later initialized as an empty list to represent the absence of data.
4. Placeholder for Future Data:
data = None
# Some code that may or may not produce data.
if data is None:
data = fetch_data_from_server()
# Fetch data if it's not available.
Here, data is set to None as a placeholder until it's populated with actual data, which is fetched from a server.
5. Removing a Value from a Dictionary:
my_dict = {'key1': 'value1', 'key2': 'value2'}
my_dict['key2'] = None # Remove 'key2' by assigning it to None.
In this example, assigning None to the key 'key2' effectively removes the associated value, indicating the absence of data for that key.
These examples demonstrate how None is used to represent missing or undefined values in Python, allowing developers to handle scenarios where the absence of data is significant.
Here's a Python script that demonstrates the use of None as described
# Initializing a Variable with None
my_variable = None
print("1. my_variable:", my_variable)
# Using None as a Default Function Return Value
def find_element_in_list(element, my_list):
for item in my_list:
if item == element:
return item
return None # If the element is not found, return None.
result = find_element_in_list(3, [1, 2, 4, 5])
print("2. Result of find_element_in_list:", result)
# Initializing an Empty List with None
my_list = None
if not my_list:
my_list = [] # Initialize my_list as an empty list.
print("3. my_list:", my_list)
# Placeholder for Future Data
data = None
# Simulating data retrieval
import random
if data is None:
data = random.randint(1, 100) # Assign a random value if data is None.
print("4. data:", data)
# Removing a Value from a Dictionary
my_dict = {'key1': 'value1', 'key2': 'value2'}
my_dict['key2'] = None # Remove 'key2' by assigning it to None.
print("5. my_dict:", my_dict)
When you run this script, you will see the output demonstrating the use of None in various scenarios. Each section is labeled with a comment to help you understand the context of the code and its output.
Compare 'None' to other Data Types
Here's a tabular comparison between None and other common data types in Python, such as 0, an empty string (""), and False. This comparison will help clarify the differences between None and False in Python, along with examples to distinguish None from other values:
Data Type | Represents | Example | Description |
---|---|---|---|
'None' | Absence of a value | 'result = None' | 'None' is used to indicate the absence of a value or missing data. |
'0' | Integer Zero | 'count = 0' | '0' represents the numeric value zero. |
Empty String | An empty text string | 'name = "" ' | An empty string represents a string with no characters. |
'False' | Boolean false | 'flag = False' | 'False' is a boolean value indicating "false" or "not true". |
Examples to Distinguish None from Other Values:
Here are some of the examples that distinguish None from other values:
Using None:
result = None
if result is None:
print("Result is not available yet.")
Here, we use None to indicate that result is not yet available.
Using 0:
count = 0
if count == 0:
print("Count is zero.")
In this case, count is explicitly set to the integer zero.
Using an Empty String:
name = ""
if name == "":
print("Name is empty.")
Here, name is an empty string with no characters.
Using False:
flag = False
if not flag:
print("Flag is False.")
flag is explicitly set to the boolean value False.
'None' is specifically used to indicate the absence of a value, making it distinct from other data types like integers, empty strings, and boolean 'False'. Understanding these differences is crucial when working with Python as it ensures that you handle each data type appropriately in your code.
Variables and NULL
Variables are used to store and manipulate data. Sometimes, you want to indicate that a variable doesn't currently have a valid or meaningful value. In Python, the None keyword is used to represent the absence of a value or to initialize variables as a way of indicating that they have no specific data.
Assigning None to Variables:
Variables can be assigned the value None to indicate that they are not holding any valid data at the moment.
This is particularly useful when you need to declare a variable before you have a suitable value to assign to it.
Illustrative Examples:
Initializing a variable with None:
my_variable = None
In this example, my_variable is initialized with None, indicating that it doesn't currently hold any specific data.
Using None for conditional checks:
result = None
if result is None:
print("Result is not available yet.")
else:
print("Result:", result)
Here, we check if result is None before displaying its value. This helps us handle cases where the result is not available.
Variable Naming Conventions: When using None to initialize variables, it's essential to follow variable naming conventions to ensure your code remains readable and maintainable:
Variable names should be descriptive and indicate their purpose. This makes it easier for you and other developers to understand the code.
If a variable is intended to be initialized with None as a placeholder, consider including a comment explaining why it's initialized this way. For example:
# Initialize count to None as a placeholder
count = None
Avoid using generic names like temp, var, or single-letter names like x for variables that will be initialized with None. Instead, use names that convey the variable's purpose or the data it will eventually hold.
Conditionals and NULL
Conditional statements (e.g., if, elif, else) are fundamental for controlling the flow of your program based on certain conditions. You can use the None keyword effectively in conditional statements to handle cases where variables or data may not have valid values. Here's how to use None in conditional statements and some illustrative code examples:
Using None in Conditional Statements:
None can be used as a value in conditional statements to check if a variable lacks a meaningful value.
It allows you to handle different cases where data might be missing or uninitialized gracefully.
Illustrative Code Examples:
Basic if Statement:
user_input = input("Enter your age: ")
result = None
if result is None:
print("Result is not available yet.")
else:
print("Result:", result)

In this example, we use an if statement to check if result is None. If it is, we print a message indicating that the result is not available yet.
Using elif to Handle Multiple Cases:
user_input = input("Enter your age: ")
if user_input is None:
print("No input provided.")
elif user_input.isdigit():
age = int(user_input)
if age < 18:
print("You are a minor.")
else:
print("You are an adult.")
else:
print("Invalid input.")

Here, we use None to check if user_input is empty. If it is, we handle that case separately. We also use elif to handle multiple conditions and nested if statements to check the age based on user input.
Handling Lists with None Elements:
data = [1, None, 3, 4, None, 6]
for item in data:
if item is None:
print("Missing data found.")
else:
print("Data:", item)
In this example, we iterate through a list and use an if statement to detect and handle missing data (represented by None) separately.
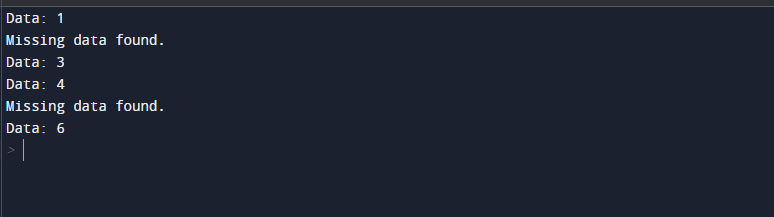
By incorporating None in your conditional statements, you can create more robust code that gracefully handles cases where data is missing or undefined, improving the reliability and readability of your programs.
Functions and NULL
You can use the None keyword in functions to indicate that a function does not return a meaningful value or to establish a default return value when no other value is explicitly returned. Here's an explanation of how None is used in functions, along with illustrative examples:
Use of None as a Default Return Value for Functions:
Functions can return None to signify that they don't produce a meaningful result.
This is useful when a function's primary purpose is to perform an action or when it may return a value under specific conditions but not always.
Creating and Calling Functions That Return None:
Function Without a Return Statement:
def greet(name):
print("Hello, " + name)
result = greet("Alice")
print("Result:", result)
In this example, the greet function does not have a return statement. It prints a greeting message but doesn't return any value. When result is printed, it will show None because the function does not explicitly return anything.
Function with a Default Return Value:
def divide(a, b):
if b == 0:
return None # Return None to indicate division by zero.
return a / b
result = divide(10, 2)
print("Result:", result) # Output: Result: 5.0
result = divide(10, 0)
print("Result:", result) # Output: Result: None

In this example, the divide function returns None when attempting to divide by zero, but it returns the result of the division when it's a valid operation.
Significance of None in Function Design:
None can be a meaningful return value when a function is designed to perform an action or task but doesn't necessarily produce a result.
It's crucial in function design to document when a function returns None so that users of the function understand its behavior.
When calling functions that return None, you can check the return value to handle special cases or errors gracefully.
Lists and NULL
You can include the None keyword in lists to represent the absence of a value or to signify missing or undefined data within the list. Here's an explanation of lists in Python, their relationship with None, and practical examples of working with lists containing None:
Lists can include None as one of their elements, just like any other data type.
None is often used in lists to indicate missing or undefined values, or as placeholders for future data.
Lists provide flexibility in handling mixed data types, including None.
Examples of Working with Lists Containing None:
Creating a List with None:
my_list = [1, None, "apple", None, 3.14]
# Printing the list
print(my_list)
# Accessing elements
print("First element:", my_list[0]) # Output: First element: 1
In this example, we create a list my_list that contains integers, strings, and None. Lists can hold elements of various types.
Iterating through a List with None:
data = [10, None, 30, None, 50]
for item in data:
if item is None:
print("Missing data found.")
else:
print("Data:", item)
This code iterates through a list data and checks for None values. When it encounters None, it prints "Missing data found." Otherwise, it prints the actual data.
Removing None from a List:
data = [1, None, 2, None, 3]
# Remove all occurrences of None from the list
data = [item for item in data if item is not None]
print(data) # Output: [1, 2, 3]
Here, we use a list comprehension to create a new list that excludes None values from the original list.
Including None in lists is a useful practice when you need to handle cases where certain data points are missing or undefined. It allows you to represent the absence of data in a structured way and work with lists that contain a mix of different data types.
Handling NULL Safely
Handling NULL in Python, represented by None in Python, is essential to ensure your code is robust and doesn't lead to unexpected errors. Here are techniques for safely handling NULL in Python code, including the use of conditional statements and try-except blocks, along with best practices:
Techniques for Safely Handling NULL Values:
Conditional Statements:
Use conditional statements (e.g., if, elif, else) to check for None before using a variable or data.
This helps you take different actions or make decisions based on whether data is present or missing.
Try-Except Blocks:
Use try and except blocks to catch and handle exceptions that may arise from NULL values.
This is particularly useful when working with external data sources or functions that might return None as an indicator of failure.
Using Conditional Statements:
data = Noneif data is None:
print("Data is missing.")
else:
print("Data:", data)
In this example, we check if data is None using an if statement before attempting to use it. This prevents errors and allows you to handle the case when data is missing gracefully.
Using Try-Except Blocks:
def divide(a, b):
try:
result = a / b
return result
except ZeroDivisionError:
print("Error: Division by zero.")
return None
result = divide(10, 0)
if result is not None:
print("Result:", result)
else:
print("No result available.")
In this code, the try block attempts the division, and if a ZeroDivisionError occurs (division by zero), it catches the exception in the except block. It prints an error message and returns None to indicate the failure.
Best Practices for Dealing with NULL Values:
Document Your Code: Clearly document when a function or variable can return None so that other developers understand the behavior.
Use Descriptive Variable Names: Use meaningful variable names that indicate when a variable might hold None.
Check for NULL Before Using: Always check for None before using a variable to avoid unexpected errors.
Avoid Implicit Conversions: Be cautious about implicitly converting None to other data types, as it can lead to unexpected behavior.
Test Thoroughly: Test your code thoroughly, including scenarios with None values, to ensure it behaves as expected.
By following these techniques and best practices, you can handle NULL in Python safely in your Python code, making it more robust and reliable.
Conclusion
NULL in Python is embodied by the 'None' keyword, representing the absence of a value or undefined data. Throughout this guide, we've learned that 'None' is a versatile tool:
Versatility: Python leverages None for initializing variables, indicating missing function return values, and as a placeholder.
Conditional Handling: None is invaluable for gracefully handling scenarios with missing data, and avoiding unexpected errors.
Function Design: Using None as a default return value communicates function behavior clearly.
Using 'None' in Python empowers you to write cleaner, more robust code. By following best practices, you'll create code that's readable, predictable, and reliable. None is your ally in building resilient solutions.
Thank you for joining us on this exploration of NULL in Python. Happy coding!
Comments