In this article, we will learn about the string processing and tips & tricks used for string processing in python.
What is String Processing?
String processing in Python refers to a set of operations or techniques that are used to manipulate, analyze and transform strings. These operations can include things like:
Extracting substrings: getting a specific part of a string
Replacing substrings: replacing one or more parts of a string with different text
Searching for substrings: finding the position of a specific substring within a string
Splitting and joining strings: dividing a string into multiple parts, or combining multiple strings into one
Formatting strings: adjusting the appearance of a string, such as aligning text or adding padding
Encoding and decoding strings: converting a string from one format to another, such as from UTF-8 to UTF-16
Regular expression: using patterns to match and extract information from strings
In Python, string processing is often done using built-in functions, such as len(), find(), replace(), split(), join(), format(), encode() and decode(). These functions can be used to perform a wide variety of string processing tasks.
Python String Processing Tips and Tricks
Here are some tips and tricks for working with strings in Python:
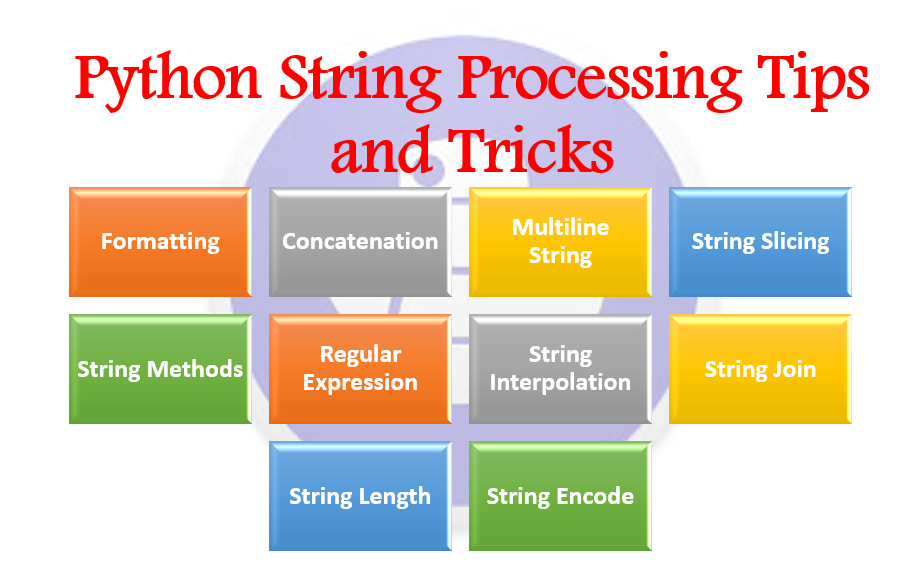
1. Formatting:
Use the .format() method to insert variables into a string. For example, "Hello, {}".format(name) will insert the value of the variable name into the string.
The .format() method in Python allows you to insert variables or expressions into a string by using placeholders, which are represented by curly braces {}. The placeholders are replaced with the values passed as arguments to the .format() method.
Here's an example of using .format() to insert a variable into a string:
Copy code
name = "Alice"
greeting = "Hello, {}!"print(greeting.format(name))
This will output the string "Hello, Alice!".
You can also use positional arguments to specify which value should replace which placeholder. In this case, the order of the arguments passed to .format() determines which placeholder they will replace.
name = "Alice"
age = 25
info = "My name is {}, and I am {} years old."
print(info.format(name, age))
This will output the string "My name is Alice, and I am 25 years old."
You can also use keyword arguments to specify which value should replace which placeholder. In this case, you need to provide the placeholders with the name of the argument.
name = "Alice"
age = 25
info = "My name is {name}, and I am {age} years old."
print(info.format(name=name, age=age))
This will output the string "My name is Alice, and I am 25 years old."
You can also use f-strings, which are string literals that are prefixed with the letter f, to embed expressions inside string literals, using {}.
name = "Alice"
age = 25
info = f"My name is {name}, and I am {age} years old."print(info)
This will output the string "My name is Alice, and I am 25 years old."
Benefits:
Clarity and readability: Using .format() to insert variables into a string can make your code more readable, as it clearly shows which values are being used in the string.
Reusability: You can use the same string with different values by calling .format() with different arguments, which can make your code more reusable.
Type safety: .format() only inserts the values passed as arguments and it will raise an exception if the number of placeholders in the string does not match the number of arguments passed to the method.
Flexibility: You can use positional arguments or keyword arguments to specify which value should replace which placeholder, providing a high degree of flexibility.
Easy to use: The .format() method is easy to use and understand, even for those who are new to Python, making it an ideal choice for beginners.
Better performance: Using .format() is faster than concatenating strings using the + operator, especially for large strings or when concatenating multiple strings.
Consistency: By using the .format() method, you can ensure that your code is consistent and easy to maintain.
Better localization: you can use .format() method with localization function, to easily format the number, date, and time according to the locales.
2. Concatenation:
Use the + operator to concatenate strings. For example, "Hello, " + name will concatenate the string "Hello, " with the value of the variable name.
Here's an example of string concatenation:
string1 = "Hello"
string2 = "world"
result = string1 + " " + string2
print(result)
This will output the string "Hello world".
You can also use the += operator to concatenate a string with another string. For example:
string1 = "Hello"
string2 = "world"
string1 += " " + string2
print(string1)
This will output the string "Hello world"
You should note that concatenation using the + operator creates a new string object and the original strings are not modified. This can be memory-intensive if you are working with large strings or need to concatenate multiple strings together. A better approach is to use the join() method of a string, which joins a list of strings together using the string as a separator.
words = ["Hello", "world"]
result = " ".join(words)
print(result)
This will output the string "Hello world".
Benefits:
Simplicity: Concatenation is a simple and straightforward way to combine strings in Python, which makes it easy to understand and use, even for those who are new to programming.
Flexibility: You can use the + operator to concatenate strings of any length, and you can concatenate any number of strings together.
Reusability: You can use the same strings in multiple concatenations, which can make your code more reusable.
Better performance: Concatenation using the += operator can be faster than using the + operator, especially when concatenating multiple strings together.
Better localization: you can use concatenation with localization function, to easily format the number, date, and time according to the locales.
Better readability: Concatenation makes it easy to understand the structure of your code and helps to keep your code readable and maintainable.
Using join() method is more efficient than using + operator if you want to concatenate a large number of strings, this is because the join() method creates a single string in memory and + operator creates a new string for each concatenation.
Using f-strings is a concise way to concatenate variables and strings, which makes it easy to understand the structure of your code and helps to keep your code readable and maintainable.
3. Multiline strings:
In Python, you can create multiline strings by using triple quotes (either single or double). This allows you to create strings that span multiple lines, without the need for explicit line continuation characters (such as \).
Here's an example of a multiline string:
multiline_string = """This is a
multiline string"""print(multiline_string)
This will output the following string:
This is a
multiline string
You can also create multiline strings using triple single quotes:
multiline_string = '''This is a
multiline string'''print(multiline_string)
This will also output the following string:
This is a
multiline string
You can use the \ character to split a string over multiple lines, but this still creates a single line string, it just makes it more readable.
multiline_string = "This is a \
multiline string"print(multiline_string)
This will also output the following string:
This is a multiline string
The benefit of using triple quotes is that it preserves the newline characters, which can be useful when creating strings that are meant to be displayed as multiple lines of text. Also, using triple quotes makes it easy to create strings that contain quotes, as you don't need to escape the quotes within the string.
Benefits:
Readability: Multiline strings can make your code more readable, as they allow you to create strings that span multiple lines, making it easy to see the structure of the string.
Formatting: Multiline strings can be useful when creating strings that are meant to be displayed as multiple lines of text, such as in the case of creating a document, an email or a markdown file.
Quotes handling: Using multiline strings allows you to create strings that contain quotes without having to escape the quotes within the string, which can make your code less prone to errors.
Code structure: You can use multiline strings to structure your code, for example to create a string that contains multiple paragraphs, or to create a template for an email or a document.
4. String slicing:
In Python, you can use square brackets [] to access individual characters or substrings (also called slices) of a string. This is called "slicing" the string.
Here's an example of accessing the first character of a string:
my_string = "Hello, world!"
first_char = my_string[0]
print(first_char)
This will output the character "H".
You can also use slicing to access a range of characters within a string. Here's an example of accessing a substring of a string:
my_string = "Hello, world!"
substring = my_string[7:12]
print(substring)
This will output the string "world".
In python, the indexing starts from 0, and the slice is defined by the starting index and the ending index separated by a colon. The slice includes the character at the starting index and all the characters up to, but not including, the character at the ending index.
You can also leave the starting or ending index blank to slice from the beginning or to the end of the string. For example, my_string[:5] will return the first five characters of the string, and my_string[7:] will return the substring starting from the 8th character to the end of the string.
You can also use negative indexing to access characters from the end of the string. For example, my_string[-1] will return the last character of the string, my_string[-3:] will return the last three characters of the string.
Slicing is a powerful and flexible feature that allows you to easily manipulate strings in Python. It can be used for tasks such as extracting substrings, removing unwanted characters, or splitting strings into smaller parts.
5. String methods:
In Python, strings have a number of built-in methods that can be used to manipulate and analyze the string data. Some common string methods include:
str.upper(): converts the string to uppercase
str.lower(): converts the string to lowercase
str.replace(old, new): replaces all occurrences of the old string with the new string
str.strip(): removes leading and trailing whitespace from the string
str.split(sep): splits the string into a list of substrings using the specified sep separator
str.find(sub): returns the index of the first occurrence of the sub string, or -1 if not found
str.count(sub): returns the number of occurrences of the sub string in the string
str.startswith(sub): returns True if the string starts with the specified sub string, False otherwise
str.endswith(sub): returns True if the string ends with the specified sub string, False otherwise
These are just a few examples of the many string methods available in Python. The official documentation provides a more complete list and more details about the usage of each method.
6. Regular expressions:
In Python, regular expressions are patterns used to match strings or parts of strings. They are often used for pattern matching with strings, or string matching, i.e "searching, editing and manipulating text."
The re module in Python provides functions for working with regular expressions. The most commonly used function is re.search(), which searches for a pattern in a string and returns a match object if found.
Here are some examples of how to use regular expressions in Python:
import re
# Search for a pattern in a string
text = "The phone number is 555-555-5555"
x = re.search("\d{3}-\d{3}-\d{4}", text)
print(x.group())
# Find all occurrences of a pattern in a string
text = "The phone number is 555-555-5555 and the emergency number is 911"
x = re.findall("\d{3}-\d{3}-\d{4}", text)
print(x)
# Substitute a pattern with a different string
text = "The phone number is 555-555-5555"
x = re.sub("\d{3}-\d{3}-\d{4}", "XXX-XXX-XXXX", text)
print(x)
Regular expressions are a powerful tool for pattern matching and text processing, but they can also be complex and difficult to read. The official documentation provides more information about the syntax and usage of regular expressions in Python.
It's also worth noting that python also provide fnmatch, glob modules for pattern matching with filenames or paths which are easier to use and understand than regular expressions.
7. String Interpolation:
String interpolation is the process of replacing placeholders in a string with their corresponding values. In Python, there are several ways to achieve string interpolation, including:
using the % operator and a tuple or dictionary of values, known as "old style" string formatting
using the format() method and curly braces {} placeholders, known as "new style" string formatting
using f-strings (formatted string literals) introduced in Python 3.6, which use curly braces {} placeholders and the letter "f" before the string.
Here are some examples of how to use string interpolation in Python:
Old style string formatting:
name = "John"
age = 30print("My name is %s and I am %d years old." % (name, age))
New style string formatting:
name = "John"
age = 30print("My name is {} and I am {} years old.".format(name, age))
f-string:
name = "John"
age = 30print(f"My name is {name} and I am {age} years old.")
It's important to notice that f-string are more efficient in terms of performance compared to the other two methods. And also it's more readable and easier to understand.
It's also worth noting that string interpolation is not recommended to be used in cases where the values to be interpolated come from untrusted sources, as it can be a security vulnerability. Instead, use parameterized queries or prepared statements to safely pass values to a database or other external service.
8. String Join:
In Python, the join() method is used to join a sequence of strings (such as a list or tuple) into a single string, using a specified delimiter. The delimiter is a string that separates each element of the sequence in the final string. The join() method is called on the delimiter string and passed the sequence as an argument.
Here's an example of how to use the join() method to join a list of strings into a single string:
words = ["Python", "is", "a", "great", "language"]
sentence = " ".join(words)
print(sentence)
# Output: "Python is a great language"
In this example, the delimiter is a single space (" "), and it's used to separate the elements of the words list in the final string.
It's also worth noting that the join() method can also be used with other types of sequence, such as tuples, and is also commonly used with generators and other iterable objects.
tuple_of_numbers = (1,2,3,4,5)
delimiter = ';'
string_of_numbers = delimiter.join(str(i) for i in tuple_of_numbers)
print(string_of_numbers) #'1;2;3;4;5'
It's also possible to use join() method on the string itself, if you want to join a list of strings into one without any delimiter, you can use empty string as a delimiter.
list_of_strings = ['Hello', 'world']
result = ''.join(list_of_strings)
print(result) # 'Helloworld'
The join() method is faster than using concatenation with the + operator, especially when working with large lists or other large sequences of strings.
9. String Length:
In Python, the length of a string can be determined by using the built-in len() function. The len() function takes an object as an argument and returns the number of items in that object. For a string, it returns the number of characters in the string. Here's an example:
string = "Hello, World!"print(len(string)) # Output: 13
In this example, the len() function is called with the string variable as an argument, and it returns the number of characters in the string, which is 13.
You can also use the built-in len() function to determine the length of a string variable without assigning it to a variable first.
print(len("Hello, World!")) # Output: 13
In python, the len() function can be used to determine the length of other types of objects as well, such as lists, tuples, and dictionaries.
numbers_list = [1,2,3,4,5]
print(len(numbers_list)) # Output: 5
When working with multi-byte characters, the string length returned by len() might not match the number of characters displayed when printed, as some characters might take up more than one byte.
10. String Encode:
In Python, the encode() method is used to encode a string into a specific encoding format. The encode() method is called on a string, and it takes an optional argument specifying the encoding to use. If no argument is provided, it defaults to UTF-8 encoding.
Here's an example of how to use the encode() method to encode a string into UTF-8:
string = "Hello, World!"
utf8_encoded = string.encode()
print(utf8_encoded) # b'Hello, World!'
In this example, the encode() method is called on the string variable and it returns a bytes object which represents the encoded string.
You can also use the encode() method to encode a string into a different encoding format, such as UTF-16 or ASCII.
string = "Hello, World!"
utf16_encoded = string.encode("utf-16")
print(utf16_encoded)
# b'\xff\xfeH\x00e\x00l\x00l\x00o\x00,\x00 \x00W\x00o\x00r\x00l\x00d\x00!\x00'
In this example, the encode() method is called with the argument "utf-16" and it returns a bytes object which represents the encoded string using UTF-16 format.
It's important to keep in mind that the encode() method raises a UnicodeEncodeError exception when it encounters a character that cannot be encoded using the specified encoding.
Conclusion
In general, string processing is a fundamental part of programming, it's often used in many applications such as web development, data analysis, text analysis, natural language processing, and many more.
The Tech Platform
Comments