Step by Step: CRUD Operation Using .NET Core Web API with Entity Framework Using SQL Server.
- The Tech Platform
- Dec 23, 2021
- 2 min read
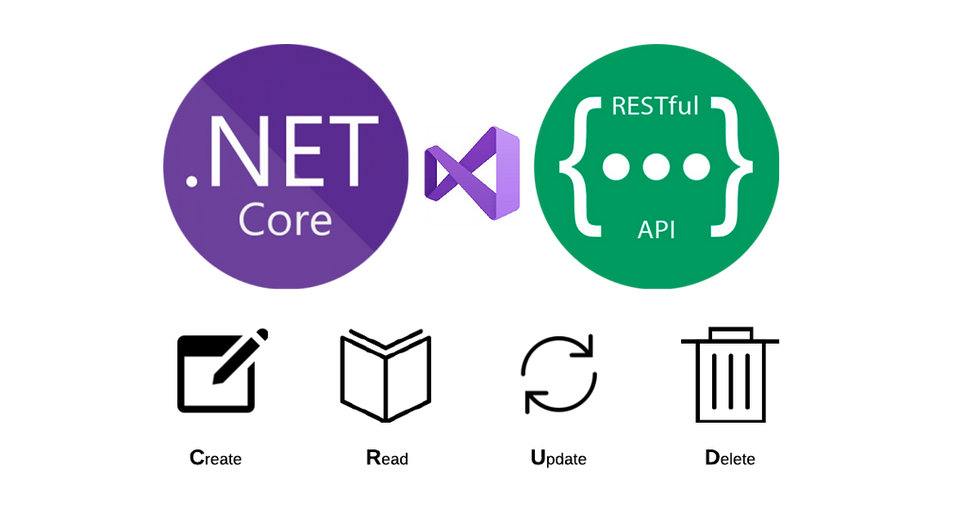
Software Requirement:
Visual Studio 2019
SQL Express
Node Package
Postman / Swagger)
What is Entity Framework :
Entity Framework is an ORM framework for .NET
ORM stands for Object Relational Mapping
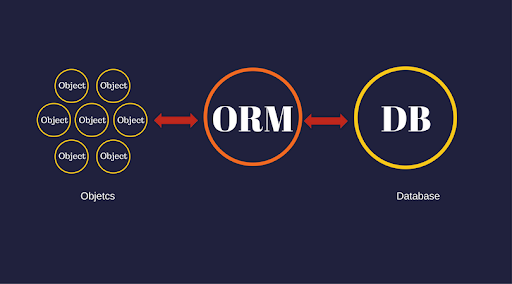
Object Relational Data Base
ORM is nothing but the bridge between the Object and Relational DataBase.
Step By Step Guide to create a CRUD Web API Using EF :
1. Create a new Project in Visual Studio Code 2019.
2. Select a ASP .NET Core Web API project. Create a new Project

3.Right click on the project name select the Manage NuGet Packages
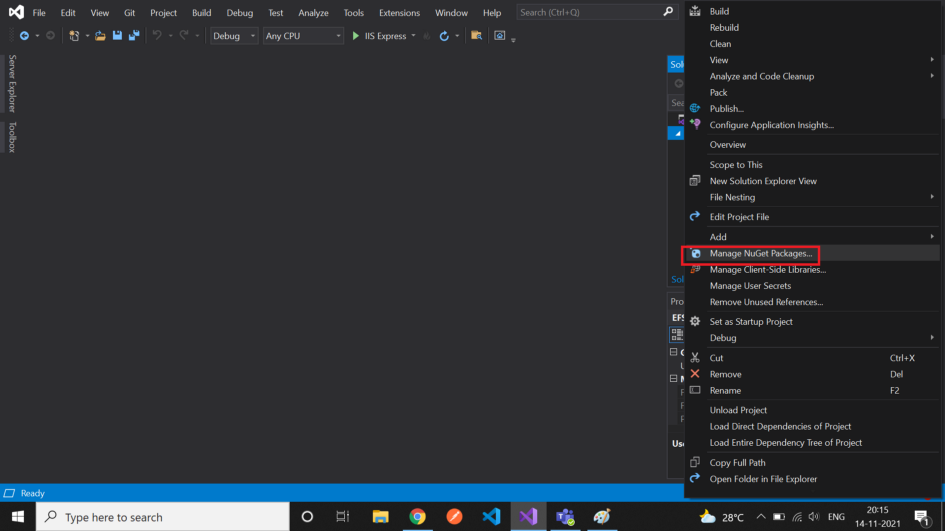
4 . Inside that select the tab Browser install the following required packages.
EntityFrameworkCore
EntityFrameworkCore.SqlServer
EntityFrameworkCore.tools
swashbuckle.AspNetCore
5. After installing all the required packages right click on the app add a new folder names Models . Inside the model create a class called Student.cs
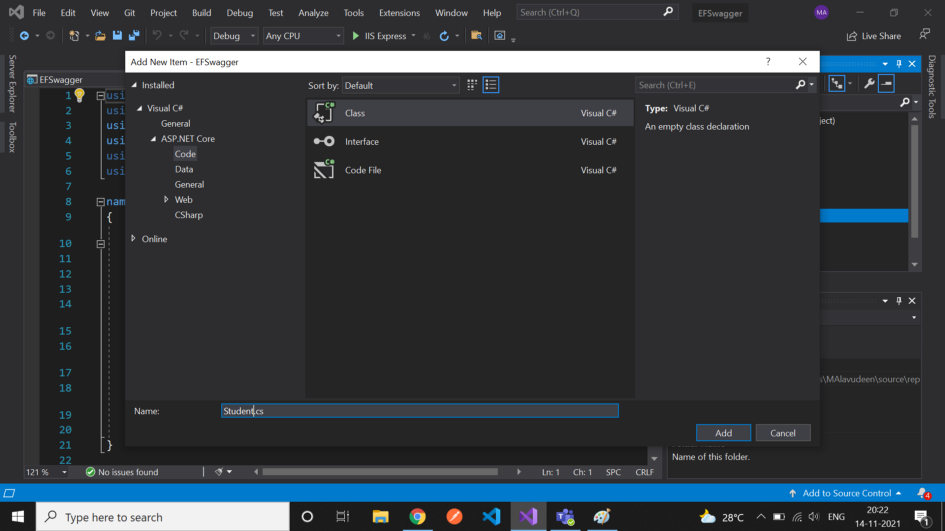
6. Inside the Student.cs write a following code
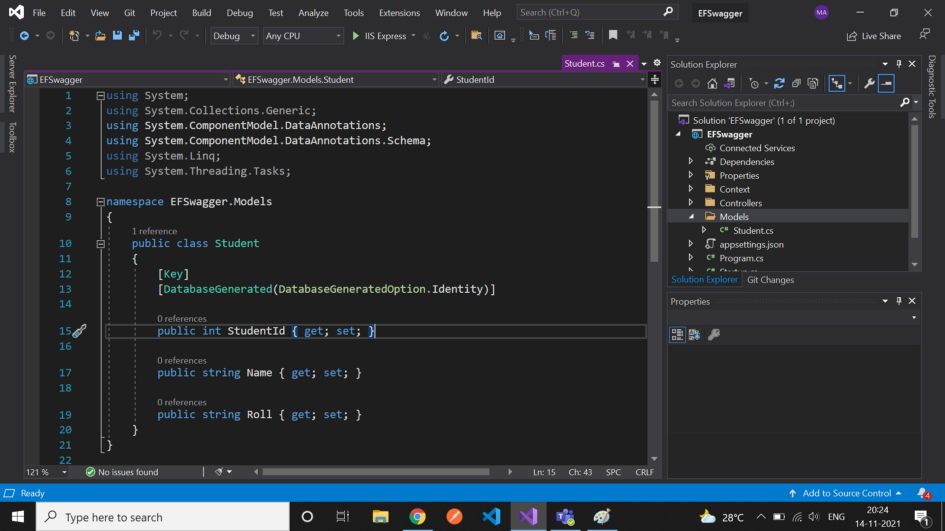
7. After that Create a new folder named as Context. Create a Contextclass.cs
8.After that go to appsetting.json . Click View→ Server Explorer (Don’t forget to connect SQL sever) click that connect icon.
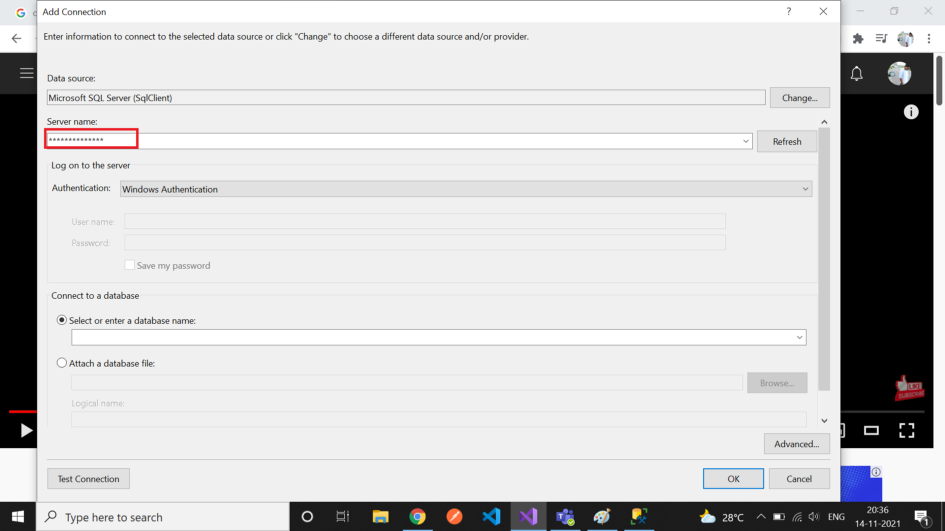
after connection inside the add ConnectionStrings.
NOTE :Write Servername inside the Data Source .
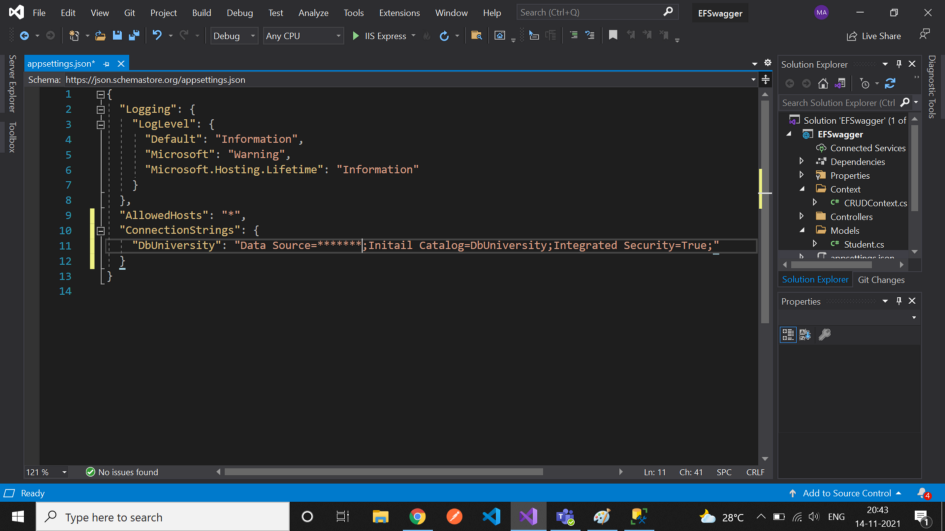
9. Next Click Startup.cs inside that write
public void ConfigureServices(IServiceCollection services)
{
services.AddMvc();
services.AddDbContext<CRUDContext>(options =>
options.UseSqlServer(Configuration.GetConnectionString
(“DbUniversity”)));
// Register Swagger
services.AddSwaggerGen(c =>
{
c.SwaggerDoc(“v1”, new OpenApiInfo
{
Title = “My API”,
Version = “v1”
});
});
}
In this CRUDContext is the context class name and DbUniversity is the Connections string name.Next add connection to Swagger
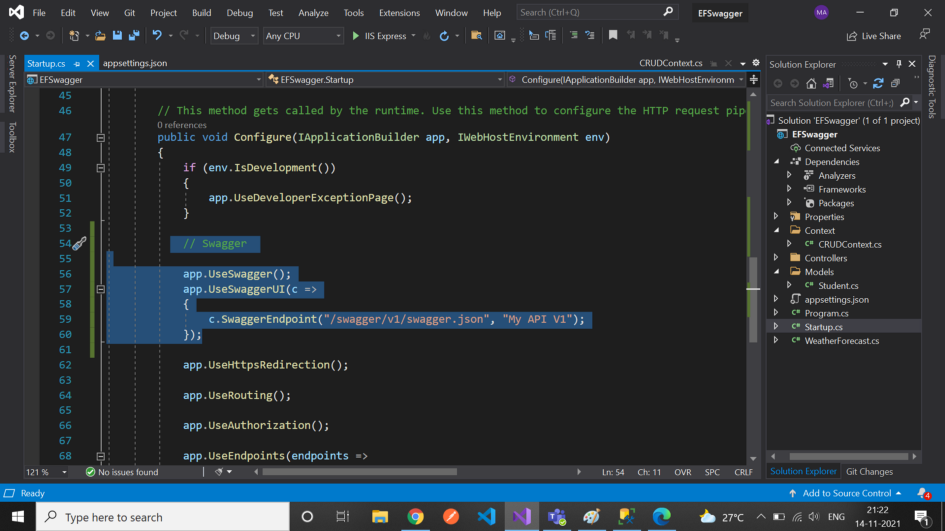
10. Then goto TOOLS → NuGet Package Manager → Package Manager Console inside that.
PM> add-migration FirstMigration
after build success ! check migration folder
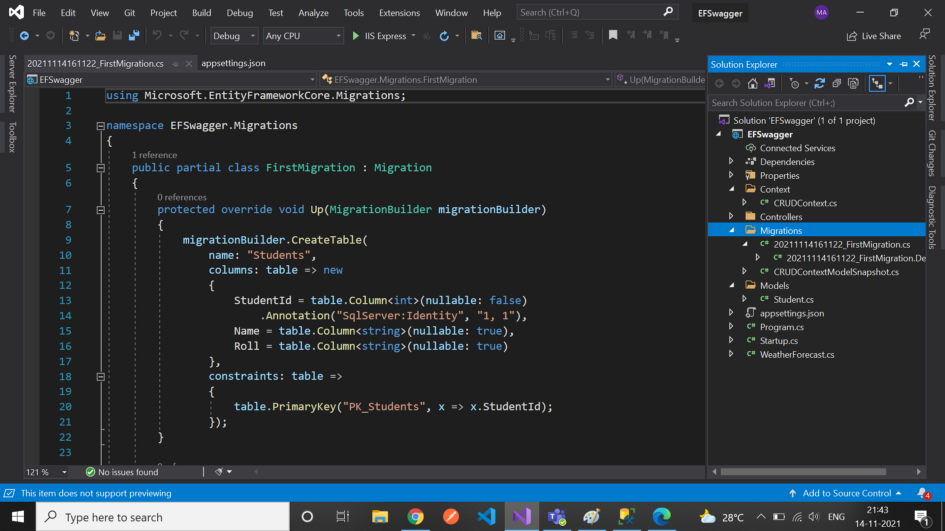
and write a cmnd PM> update-database
after successfully build check database in SQLexpress .
11 . Inside Controller Folder create a class named Students.cs .
Note: Name must be same as model name
using EFSwagger.Context;
using EFSwagger.Models;
using Microsoft.AspNetCore.Mvc;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
// For more information on enabling Web API for empty projects, visit https://go.microsoft.com/fwlink/?LinkID=397860
namespace EFSwagger.Controllers
{
[Route(“api/[controller]”)]
[ApiController]
public class StudentsController : ControllerBase
{
private readonly CRUDContext _CRUDContext;
public StudentsController(CRUDContext CRUDContext)
{
_CRUDContext = CRUDContext;
}
// GET: api/<StudentsController>
[HttpGet]
public IEnumerable<Student> Get()
{
return _CRUDContext.Students;
}
// GET api/<StudentsController>/5
[HttpGet(“{id}”,Name = “Get”)]
public Student Get(int id)
{
return _CRUDContext.Students.SingleOrDefault(x => x.StudentId == id);
}
// POST api/<StudentsController>
[HttpPost]
public void Post([FromBody] Student student)
{
_CRUDContext.Students.Add(student);
_CRUDContext.SaveChanges();
}
// PUT api/<StudentsController>/5
[HttpPut(“{id}”)]
public void Put( [FromBody] Student student)
{
_CRUDContext.Students.Update(student);
_CRUDContext.SaveChanges();
}
// DELETE api/<StudentsController>/5
[HttpDelete(“{id}”)]
public void Delete(int id)
{
var item = _CRUDContext.Students.FirstOrDefault(x => x.StudentId
== id);
if (item !=null)
{
_CRUDContext.Students.Remove(item);
_CRUDContext.SaveChanges();
}
}
}
}
After finishing the controller part don’t forget to change the launching path
it’s in solution explorer → Properties → launchSetting.json in that change the launchURL to swagger for both profile and appname . after run the program its shows the resultant output in swagger .
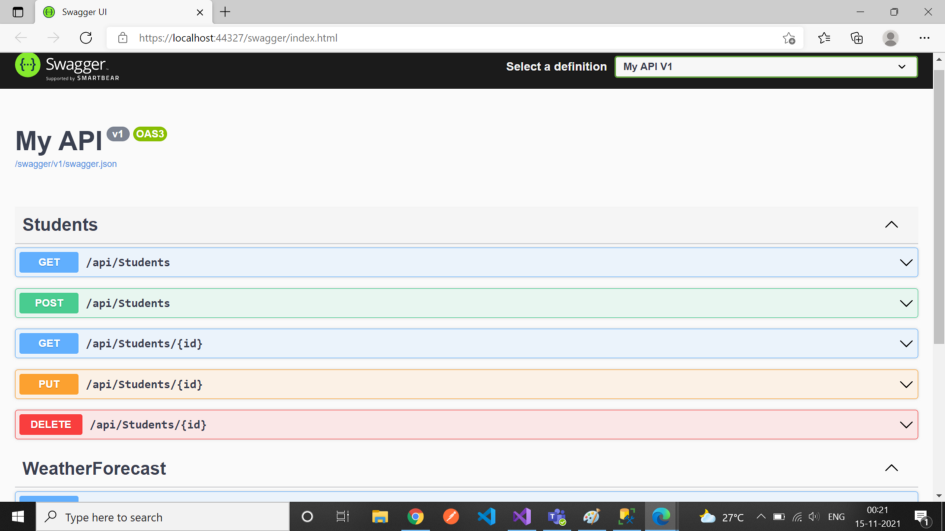
Source: Medium - Yahiya
The Tech Platform
Comments