++i and i++ both increment the value of i by 1 but in a different way. If ++ precedes the variable, it is called pre-increment operator and it comes after a variable, it is called post-increment operator.
The main difference is the order of operations between the increment of the variable and the value the operator returns. So ++i returns the value after it is incremented, while i++ return the value before it is incremented. At the end, in both cases the i will have its value incremented.
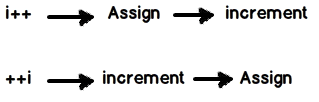
Post-increment i++
i++ is post increment operator, which increment the value of i after execution, and it provides the current value of i first and then increment i.
the original value is loaded into memory, then increment the variable
the value of the expression is the original value
Pre-increment ++i
++i is pre-increment operator. Which increment the value of i during the execution, and it provides the incremented value of i.
increment the variable
the value of the expression is the final value
Example of Post-increment and Pre-increment:
import java.io.*;
class GFG {
public static void main(String[] args)
{
// initialize i
int i = 0;
System.out.println("Post-Increment");
// i values is incremented to 1 after returning
// current value i.e; 0
System.out.println(i++);
// initialized to 0
int j = 0;
System.out.println("Pre-Increment");
// j is incremented to 1 and then it's value is
// returned
System.out.println(++j);
}
}
Output:
Post-Increment
0
Pre-Increment
1
The Tech Platform
Comments