The program works by iterating over each row and column of the pattern and checking if the current position is on the edge of the pattern, on the diagonal, or on the reverse diagonal. If it is, it prints a *, otherwise, it prints a space.
Algorithm
Here are the steps for the program:
STEP 1: Start
STEP 2: Initialize n to the desired size of the pattern
STEP 3: Start a loop over the rows of the pattern, with i starting at 1 and ending at n
STEP 4: Within the row loop, start a loop over the columns of the pattern, with j starting at 1 and ending at n
STEP 5: Check if the current position is on the edge of the pattern, on the diagonal, or on the reverse diagonal
STEP 6: If the position is on the edge or on the diagonal, print a *
STEP 7: If the position is not on the edge or on the diagonal, print a space
STEP 8: End the column loop
STEP 9: Print a newline to start a new row
STEP 10: End the row loop
STEP 11: End the program.
Code:
#include <iostream>
using namespace std;
int main() {
int n = 15; // change this to set the size of the pattern
// print pattern
for (int i = 1; i <= n; i++) {
for (int j = 1; j <= n; j++) {
if (i == 1 || i == n || j == 1 || j == n || i == j || i == n - j + 1) {
cout << "*";
} else {
cout << " ";
}
}
cout << endl;
}
return 0;
}
Output:
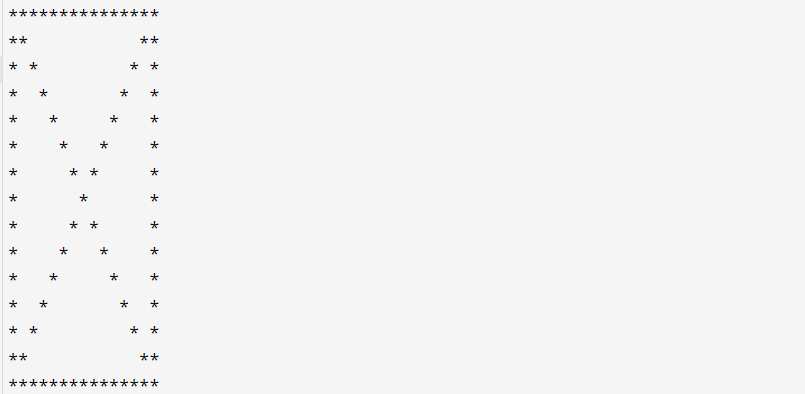
コメント